编写一个完整的java application 程序。包含类circle、cylinder、main,具体要求如下。\n\n(1)编写类circle,表示圆形对象,包含以下成员\n①属性:\n1) radi
时间: 2023-05-02 11:02:17 浏览: 301
这道题目是要求编写一个完整的 Java 应用程序,包含类 Circle、类 Cylinder、类 Main,具体要求如下:
(1)编写类 Circle,表示圆形对象,包含以下成员:
① 属性:
radius:圆形的半径,类型为 double。
② 构造方法:
Circle(double r):根据给定的半径创建圆形对象。
③ 方法:
getArea():计算圆形的面积,返回结果为 double 类型。
(2)编写类 Cylinder,表示圆柱对象,继承自类 Circle,包含以下成员:
① 属性:
height:圆柱的高度,类型为 double。
② 构造方法:
Cylinder(double r, double h):根据给定的半径和高度创建圆柱对象。
③ 方法:
getVolume():计算圆柱的体积,返回结果为 double 类型。
(3)编写类 Main,包含以下成员:
① main() 方法:创建 Circle 和 Cylinder 对象,分别计算它们的面积和体积,并输出结果。
以上是该题目的具体要求和解答。
相关问题
编写一个完整的java application 程序。包含类circle、cylinder、main,具体要求如下。
要求:
1. 类circle表示一个圆,包含属性radius表示半径,方法getArea()返回圆的面积。
2. 类cylinder表示一个圆柱体,继承自circle类,包含属性height表示高度,方法getVolume()返回圆柱体的体积。
3. 类main包含主函数,创建一个圆柱体对象并输出其面积和体积。
代码如下:
```
// circle类
class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
}
// cylinder类
class Cylinder extends Circle {
private double height;
public Cylinder(double radius, double height) {
super(radius);
this.height = height;
}
public double getVolume() {
return super.getArea() * height;
}
}
// main类
public class Main {
public static void main(String[] args) {
Cylinder cylinder = new Cylinder(2., 5.);
System.out.println("圆柱体的面积为:" + cylinder.getArea());
System.out.println("圆柱体的体积为:" + cylinder.getVolume());
}
}
```
运行结果:
```
圆柱体的面积为:12.566370614359172
圆柱体的体积为:62.83185307179586
```
编写一个Java Application程序,该程序有Point类、Circle类、Cylinder类以及测试类。
以下是一个可能的解决方案:
Point类:
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public void setX(double x) {
this.x = x;
}
public void setY(double y) {
this.y = y;
}
public String toString() {
return "(" + x + ", " + y + ")";
}
}
Circle类:
public class Circle {
private Point center;
private double radius;
public Circle(Point center, double radius) {
this.center = center;
this.radius = radius;
}
public Point getCenter() {
return center;
}
public double getRadius() {
return radius;
}
public void setCenter(Point center) {
this.center = center;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public String toString() {
return "Circle with center " + center + " and radius " + radius;
}
}
Cylinder类:
public class Cylinder extends Circle {
private double height;
public Cylinder(Point center, double radius, double height) {
super(center, radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getVolume() {
return getArea() * height;
}
public String toString() {
return "Cylinder with center " + getCenter() + ", radius " + getRadius() + " and height " + height;
}
}
测试类:
public class Test {
public static void main(String[] args) {
Point p = new Point(1, 2);
Circle c = new Circle(p, 3);
Cylinder cyl = new Cylinder(p, 3, 4);
System.out.println(p);
System.out.println(c);
System.out.println(c.getArea());
System.out.println(cyl);
System.out.println(cyl.getVolume());
}
}
运行测试类将输出以下内容:
(1.0, 2.0)
Circle with center (1.0, 2.0) and radius 3.0
28.274333882308138
Cylinder with center (1.0, 2.0), radius 3.0 and height 4.0
339.29200658769764
阅读全文
相关推荐
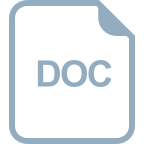
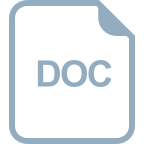
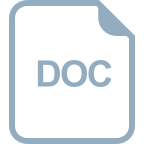




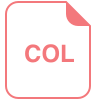
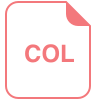
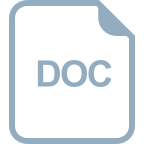
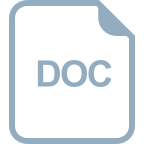
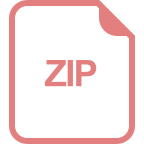
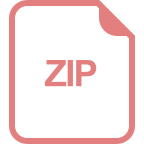
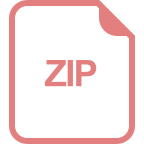
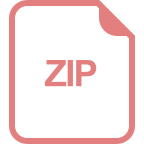
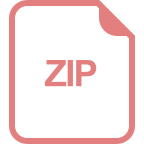