springboot+vue 上传图片,将图片存储到数据库
时间: 2023-11-24 13:05:17 浏览: 224
将图片存储到数据库并不是一个好的做法,因为数据库存储的是结构化数据,而图片是二进制数据,会占用大量的数据库存储空间,降低数据库的性能。更好的做法是将图片存储到文件系统或者对象存储中,然后在数据库中存储图片的路径或者文件名。
下面是一个简单的上传图片并将图片路径存储到数据库的示例:
1.前端页面使用Vue上传图片
```html
<template>
<div>
<input type="file" @change="handleFileChange">
<button @click="uploadImage">上传图片</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null
}
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
uploadImage() {
let formData = new FormData();
formData.append('file', this.file);
axios.post('http://localhost:8080/upload', formData)
.then(response => {
// 上传成功后,将图片路径存储到数据库
let imagePath = response.data.imagePath;
// ...
})
.catch(error => {
console.log(error);
});
}
}
}
</script>
```
2.后端使用Spring Boot接收并处理上传的图片
```java
@RestController
public class UploadController {
@Autowired
private ImageService imageService;
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 保存图片到文件系统或者对象存储
String imagePath = imageService.saveImage(file);
// 将图片路径存储到数据库
// ...
return ResponseEntity.ok().body(new UploadResponse(imagePath));
} catch (Exception e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
public class UploadResponse {
private String imagePath;
// getter and setter
}
@Service
public class ImageService {
public String saveImage(MultipartFile file) throws IOException {
// 保存图片到文件系统或者对象存储
// ...
return imagePath;
}
}
```
阅读全文
相关推荐
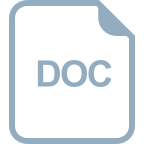
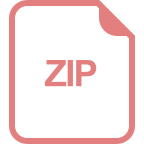
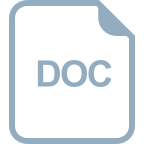
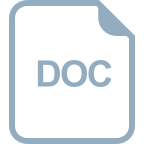
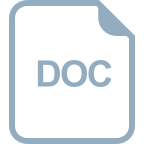
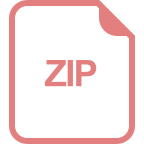
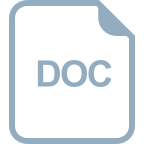
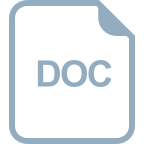
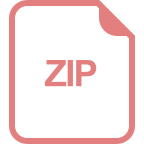
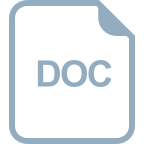
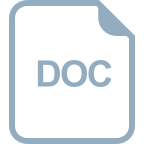
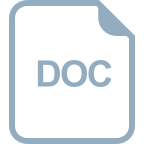
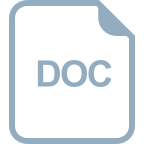


