在使用验证框架的情况下,该如何写实现类和controller
时间: 2024-04-15 11:27:02 浏览: 101
在使用验证框架时,您需要编写实体类、验证注解、实现类和控制器。下面是一个示例:
1.体类 `RoublePersonnelPool.java:
```java
import javax.validation.constraints.NotBlank;
public class RoublePersonnelPool {
@NotBlank(message = "序号不能为空")
private String numbleId;
@NotBlank(message = "隶属单位不能为空")
private String roubleUnit;
@NotBlank(message = "部门不能为空")
private String roubleBumen;
@NotBlank(message = "人员名称不能为空")
private String roubleName;
@NotBlank(message = "编号不能为空")
private String roubleID;
// 省略 getter 和 setter 方法
}
```
在上述示例中,我们使用了`@NotBlank`注解来标记字段属性为非空字符串。
2. 实现类 `RoublePersonnelPoolServiceImpl.java`:
```java
import org.springframework.stereotype.Service;
import org.springframework.validation.annotation.Validated;
@Service
@Validated
public class RoublePersonnelPoolServiceImpl implements RoublePersonnelPoolService {
@Override
public RoublePersonnelPool saveRoublePersonnelPool(@Valid RoublePersonnelPool roublePersonnelPool) {
// 执行保存操作
return roublePersonnelPool;
}
}
```
在上述示例中,我们在实现类上添加了`@Validated`注解,这样Spring会自动对方法中使用了验证注解的参数进行校验。
3. 控制器 `RoublePersonnelPoolController.java`:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
@RestController
public class RoublePersonnelPoolController {
private final RoublePersonnelPoolService roublePersonnelPoolService;
@Autowired
public RoublePersonnelPoolController(RoublePersonnelPoolService roublePersonnelPoolService) {
this.roublePersonnelPoolService = roublePersonnelPoolService;
}
@PostMapping("/rouble-personnel-pool")
public ResponseEntity<?> saveRoublePersonnelPool(@Valid @RequestBody RoublePersonnelPool roublePersonnelPool, BindingResult result) {
if (result.hasErrors()) {
// 如果验证不通过,返回错误信息
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body(result.getAllErrors());
}
// 执行保存操作
RoublePersonnelPool savedRoublePersonnelPool = roublePersonnelPoolService.saveRoublePersonnelPool(roublePersonnelPool);
// 返回保存后的结果
return ResponseEntity.ok(savedRoublePersonnelPool);
}
}
```
在上述示例中,我们使用了`@Valid`注解来标记需要进行验证的参数,同时还使用了`BindingResult`对象来获取验证结果。如果验证不通过,我们可以将错误信息返回给客户端。
请注意,上述代码示例仅供参考,您可能需要根据您的具体需求进行适当的修改。另外,还需要根据您使用的验证框架进行相应的配置和依赖管理。
阅读全文
相关推荐
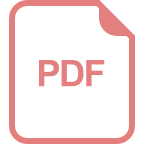
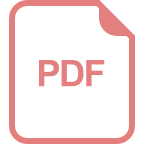
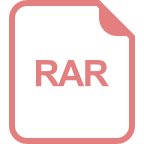
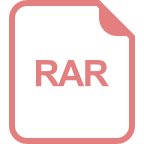
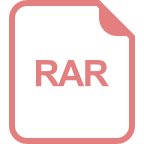
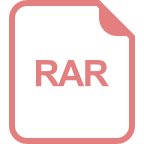
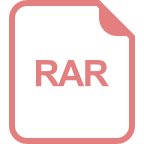
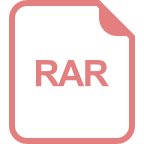
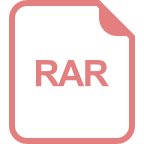
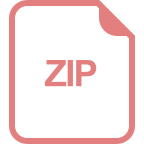
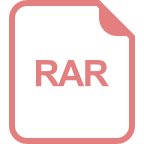
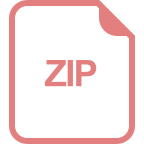
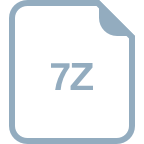
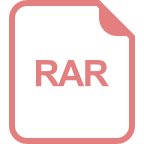
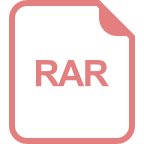
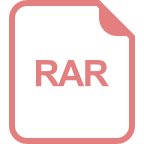
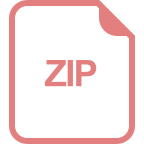
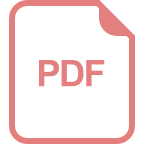