编写用多线程模拟火车站售票大厅的情形,包括:添加顾客、顾客离开、增加售票窗口、关闭售票窗口功能
时间: 2023-07-10 07:21:10 浏览: 88
好的,以下是一个简单的多线程模拟火车站售票大厅的程序:
```python
import threading
import time
import random
class TrainStation:
def __init__(self):
self.customers = []
self.tickets = 100
self.ticket_windows = 5
self.open = True
def add_customer(self):
self.customers.append("Customer {}".format(len(self.customers)+1))
print("Added a customer. Total customers: {}".format(len(self.customers)))
def remove_customer(self):
if len(self.customers) > 0:
self.customers.pop(0)
print("Removed a customer. Total customers: {}".format(len(self.customers)))
def sell_ticket(self):
if self.tickets > 0:
self.tickets -= 1
print("Sold a ticket. Tickets left: {}".format(self.tickets))
def add_window(self):
self.ticket_windows += 1
print("Added a ticket window. Total windows: {}".format(self.ticket_windows))
def remove_window(self):
if self.ticket_windows > 0:
self.ticket_windows -= 1
print("Removed a ticket window. Total windows: {}".format(self.ticket_windows))
def close_station(self):
self.open = False
print("Train station closed.")
def customer_arrive(station):
while station.open:
time.sleep(random.randint(1,5))
station.add_customer()
def customer_leave(station):
while station.open:
time.sleep(random.randint(5,10))
station.remove_customer()
def sell_ticket(station):
while station.open:
time.sleep(1)
if len(station.customers) > 0 and station.ticket_windows > 0:
station.sell_ticket()
def add_window(station):
while station.open:
time.sleep(random.randint(10,20))
station.add_window()
def remove_window(station):
while station.open:
time.sleep(random.randint(10,20))
station.remove_window()
def close_station(station):
time.sleep(60)
station.close_station()
if __name__ == "__main__":
station = TrainStation()
threads = [
threading.Thread(target=customer_arrive, args=(station,)),
threading.Thread(target=customer_leave, args=(station,)),
threading.Thread(target=sell_ticket, args=(station,)),
threading.Thread(target=add_window, args=(station,)),
threading.Thread(target=remove_window, args=(station,)),
threading.Thread(target=close_station, args=(station,))
]
for t in threads:
t.start()
for t in threads:
t.join()
print("Simulation finished.")
```
这个程序模拟了火车站售票大厅的场景,包括添加顾客、顾客离开、增加售票窗口、关闭售票窗口功能。在主函数中,我们创建了一个 TrainStation 对象,并创建了多个线程来模拟不同的行为。每个线程都会在一定的时间间隔内执行相应的操作,直到火车站关闭。最后,我们使用 join() 方法等待所有线程执行完毕,然后输出 "Simulation finished."。
阅读全文
相关推荐
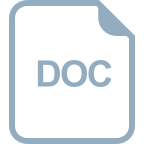
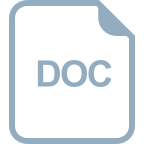
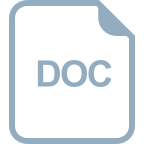


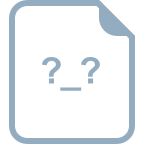


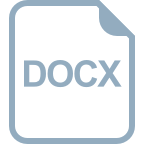
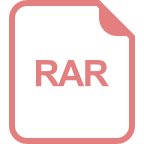
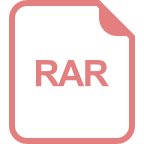







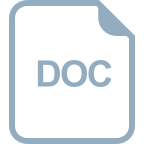