使用visual studio code 制作一个平面动画,设置canvas为400,400。将背景设置为黑色。在画布正中间放置一个直径为70的球,静止,代表太阳,颜色为红色。同圆心,半径150,放置一个直径30的绿色球代表地球,围绕着太阳旋转,速度合理即可。键盘s键可以暂停,g键可以继续。地球在90度,180度,270度会改变颜色,颜色自定。放置一个直径为10的黄色球代表月亮围绕地球旋转
时间: 2024-04-07 15:31:49 浏览: 52
好的,我可以帮你解决这个问题。首先,你需要在Visual Studio Code中安装一个插件来启用Canvas。在你的工作区中创建一个新的HTML文件,并将其命名为index.html。在文件头部添加以下代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Canvas Animation</title>
<style>
canvas {
background-color: black;
display: block;
margin: 0 auto;
margin-top: 50px;
border: 1px solid white;
}
</style>
</head>
<body>
<canvas id="myCanvas" width="400" height="400"></canvas>
<script src="app.js"></script>
</body>
</html>
```
这里我们定义了一个canvas元素,宽度和高度都为400。我们还定义了一个样式,将背景颜色设置为黑色,并在画布上添加一个白色的边框。
接下来,我们需要在项目中创建一个JavaScript文件来操作Canvas。在你的工作区中创建一个新的JavaScript文件,并将其命名为app.js。在文件头部添加以下代码:
```javascript
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
var sun = {
x: canvas.width/2,
y: canvas.height/2,
radius: 70,
color: "red",
};
var earth = {
x: canvas.width/2 + 150,
y: canvas.height/2,
radius: 30,
color: "green",
theta: 0,
speed: 0.01,
};
var moon = {
x: earth.x + 50,
y: earth.y,
radius: 10,
color: "yellow",
theta: 0,
speed: 0.05,
};
var isPaused = false;
function drawSun() {
ctx.beginPath();
ctx.arc(sun.x, sun.y, sun.radius, 0, 2*Math.PI);
ctx.fillStyle = sun.color;
ctx.fill();
}
function drawEarth() {
ctx.beginPath();
ctx.arc(earth.x, earth.y, earth.radius, 0, 2*Math.PI);
ctx.fillStyle = earth.color;
ctx.fill();
}
function drawMoon() {
ctx.beginPath();
ctx.arc(moon.x, moon.y, moon.radius, 0, 2*Math.PI);
ctx.fillStyle = moon.color;
ctx.fill();
}
function updateEarth() {
earth.x = canvas.width/2 + 150*Math.cos(earth.theta);
earth.y = canvas.height/2 + 150*Math.sin(earth.theta);
earth.theta += earth.speed;
if (earth.theta >= 2*Math.PI) {
earth.theta = 0;
}
if (earth.theta >= Math.PI/2 && earth.theta < Math.PI) {
earth.color = "blue";
} else if (earth.theta >= Math.PI && earth.theta < 3*Math.PI/2) {
earth.color = "purple";
} else {
earth.color = "green";
}
}
function updateMoon() {
moon.x = earth.x + 50*Math.cos(moon.theta);
moon.y = earth.y + 50*Math.sin(moon.theta);
moon.theta += moon.speed;
if (moon.theta >= 2*Math.PI) {
moon.theta = 0;
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSun();
drawEarth();
drawMoon();
updateEarth();
updateMoon();
if (!isPaused) {
requestAnimationFrame(draw);
}
}
draw();
document.addEventListener("keydown", function(event) {
if (event.key === "s") {
isPaused = true;
} else if (event.key === "g") {
isPaused = false;
requestAnimationFrame(draw);
}
});
```
这里我们首先获取了canvas元素和其上下文对象ctx。然后我们定义了三个对象,分别表示太阳,地球和月亮的属性。我们还定义了一个布尔变量isPaused,以便在按下s键时暂停动画。我们还定义了四个函数,分别用于绘制太阳,地球,月亮和更新地球的位置。最后,我们定义了一个函数draw,用于绘制整个场景。我们还添加了一个事件监听器,以便在按下s键时暂停动画,在按下g键时继续动画。
现在你可以打开index.html文件,你将看到在画布正中央有一个红色的太阳,一个围绕太阳旋转的绿色地球,以及一个围绕地球旋转的黄色月亮。在90度,180度和270度时,地球的颜色将改变为蓝色,紫色和绿色。你可以按下s键暂停动画,按下g键恢复动画。
相关推荐
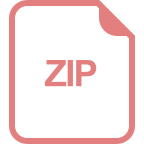
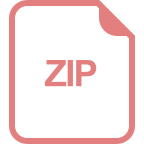
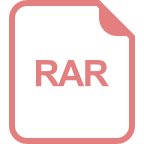
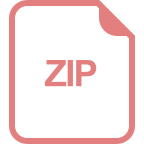
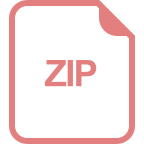
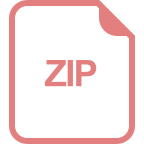
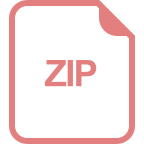
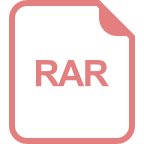
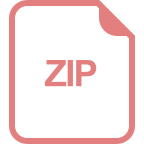
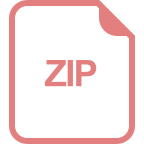
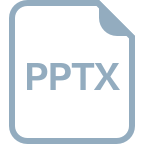
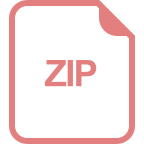
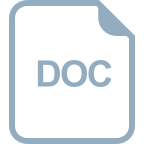
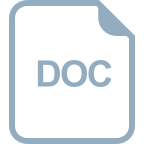
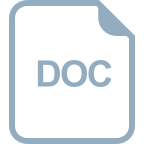
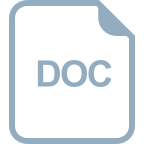
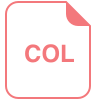
