写一段python程序,读取txt文件中的数据并将其转化为二进制
时间: 2024-04-29 19:25:10 浏览: 119
好的,以下是Python程序示例:
```python
with open('data.txt', 'r') as file:
data = file.read()
binary_data = ''.join(format(ord(char), '08b') for char in data)
print(binary_data)
```
这段程序可以读取名为"data.txt"的文本文件中的数据,并将其转换为二进制格式。请确保"data.txt"文件与Python程序在同一目录中,或者在文件路径中指定正确的位置。
相关问题
要求将一个文本文件(20031134),用python读入后,将其转化为二进制文件存放;同时再用python读入这个二进制文件,以文本文件的形式写入磁盘。
要将一个文本文件(20031134)用Python读入后转化为二进制文件保存,然后再将这个二进制文件读入并以文本文件的形式写入磁盘,可以按照以下步骤进行:
1. 读取文本文件并将其内容转换为二进制形式保存。
2. 读取二进制文件并将其内容转换回文本形式保存。
以下是具体的Python代码示例:
```python
# 读取文本文件并将其内容转换为二进制形式保存
def text_to_binary(input_file, output_file):
with open(input_file, 'r', encoding='utf-8') as text_file:
text_content = text_file.read()
binary_content = text_content.encode('utf-8')
with open(output_file, 'wb') as binary_file:
binary_file.write(binary_content)
# 读取二进制文件并将其内容转换回文本形式保存
def binary_to_text(input_file, output_file):
with open(input_file, 'rb') as binary_file:
binary_content = binary_file.read()
text_content = binary_content.decode('utf-8')
with open(output_file, 'w', encoding='utf-8') as text_file:
text_file.write(text_content)
# 示例使用
input_text_file = '20031134.txt'
output_binary_file = '20031134.bin'
output_text_file = '20031134_converted.txt'
# 将文本文件转换为二进制文件
text_to_binary(input_text_file, output_binary_file)
# 将二进制文件转换回文本文件
binary_to_text(output_binary_file, output_text_file)
```
这段代码首先定义了两个函数:
1. `text_to_binary`:读取文本文件内容并将其编码为UTF-8格式的二进制数据,然后写入到指定的二进制文件中。
2. `binary_to_text`:读取二进制文件内容并将其解码为UTF-8格式的文本数据,然后写入到指定的文本文件中。
通过这两个函数,可以实现文本文件与二进制文件之间的相互转换。
将二进制数据文件转化成十六进制文件出力的python代码
要将二进制数据文件转换成十六进制文件,可以使用Python的内置库如`binascii`或直接使用字符串操作。以下是一个简单的例子,展示了如何将二进制文件内容转换为十六进制字符串,并将结果写入新的文件中。
```python
def binary_to_hex(input_file_path, output_file_path):
# 打开二进制文件进行读取
with open(input_file_path, 'rb') as binary_file:
# 读取所有内容
binary_data = binary_file.read()
# 将二进制数据转换为十六进制表示
hex_data = binary_data.hex()
# 打开输出文件准备写入
with open(output_file_path, 'w') as hex_file:
# 将十六进制数据写入文件
hex_file.write(hex_data)
# 使用示例
input_file = 'example.bin' # 假设这是输入的二进制文件名
output_file = 'example.hex' # 输出的十六进制文件名将会被创建
binary_to_hex(input_file, output_file)
```
这段代码定义了一个函数`binary_to_hex`,它接受输入文件和输出文件的路径作为参数。使用`'rb'`模式以二进制形式打开输入文件,并读取其内容。然后使用`hex()`方法将二进制数据转换为十六进制字符串。最后,以文本模式打开输出文件,并将十六进制字符串写入其中。
确保在运行此代码之前,输入文件`input_file_path`存在且具有可读权限,同时程序具有写入输出文件`output_file_path`所在目录的权限。
阅读全文
相关推荐



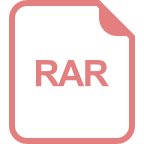









