python编写一个脚本,实现远程控制功能:命令执行、文件上传和文件下载
时间: 2024-05-16 11:18:01 浏览: 80
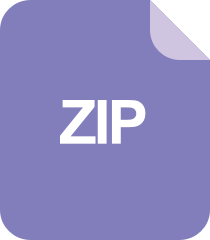
diffcp:将文件从一个文件夹复制到输出文件夹的 Python 脚本,仅当它们不存在于另一个文件夹中时
实现远程控制功能需要先建立服务器和客户端之间的连接,可以使用Python的socket模块实现。下面是一个简单的脚本示例,实现了命令执行、文件上传和文件下载三项功能。
服务端代码:
```python
import socket
import subprocess
import os
SERVER_HOST = '0.0.0.0'
SERVER_PORT = 9999
BUFFER_SIZE = 4096
def execute_command(command):
try:
output = subprocess.check_output(command, shell=True)
except Exception as e:
output = str(e).encode()
return output
def send_file(conn, file_path):
with open(file_path, 'rb') as f:
data = f.read(BUFFER_SIZE)
while data:
conn.send(data)
data = f.read(BUFFER_SIZE)
def receive_file(conn, file_path):
with open(file_path, 'wb') as f:
data = conn.recv(BUFFER_SIZE)
while data:
f.write(data)
data = conn.recv(BUFFER_SIZE)
def handle_connection(conn, addr):
print(f'[*] New connection from {addr[0]}:{addr[1]}')
while True:
data = conn.recv(BUFFER_SIZE)
if not data:
break
command = data.decode().strip()
if command.startswith('cd'):
os.chdir(command[3:])
output = b''
elif command.startswith('upload'):
file_path = command[7:]
receive_file(conn, file_path)
output = b'File uploaded successfully'
elif command.startswith('download'):
file_path = command[9:]
send_file(conn, file_path)
output = b''
else:
output = execute_command(command)
conn.send(output)
conn.close()
def main():
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((SERVER_HOST, SERVER_PORT))
s.listen(5)
print(f'[*] Listening on {SERVER_HOST}:{SERVER_PORT}')
while True:
conn, addr = s.accept()
handle_connection(conn, addr)
if __name__ == '__main__':
main()
```
客户端代码:
```python
import socket
import os
SERVER_HOST = '127.0.0.1'
SERVER_PORT = 9999
BUFFER_SIZE = 4096
def execute_command(command):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((SERVER_HOST, SERVER_PORT))
s.send(command.encode())
output = b''
data = s.recv(BUFFER_SIZE)
while data:
output += data
data = s.recv(BUFFER_SIZE)
s.close()
return output.decode()
def send_file(file_path):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((SERVER_HOST, SERVER_PORT))
s.send(f'upload {file_path}'.encode())
with open(file_path, 'rb') as f:
data = f.read(BUFFER_SIZE)
while data:
s.send(data)
data = f.read(BUFFER_SIZE)
s.close()
def receive_file(file_path):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((SERVER_HOST, SERVER_PORT))
s.send(f'download {file_path}'.encode())
with open(file_path, 'wb') as f:
data = s.recv(BUFFER_SIZE)
while data:
f.write(data)
data = s.recv(BUFFER_SIZE)
s.close()
def main():
while True:
command = input('> ')
if command == 'exit':
break
elif command.startswith('upload'):
file_path = command[7:]
if os.path.exists(file_path):
send_file(file_path)
print('File uploaded successfully')
else:
print(f'{file_path} does not exist')
elif command.startswith('download'):
file_path = command[9:]
receive_file(file_path)
print('File downloaded successfully')
else:
output = execute_command(command)
print(output)
if __name__ == '__main__':
main()
```
使用方法:
1. 在服务端计算机上运行服务端脚本
2. 在客户端计算机上运行客户端脚本,并输入服务端计算机的IP地址和端口号
3. 在客户端上输入命令,可以执行远程命令、上传文件和下载文件。例如,执行`ls`命令,上传`test.txt`文件,下载`example.txt`文件等。
阅读全文
相关推荐
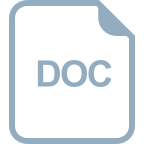
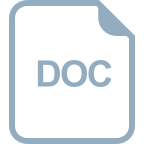


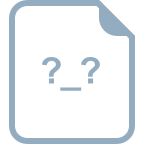
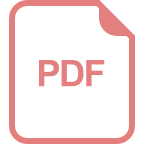
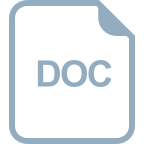
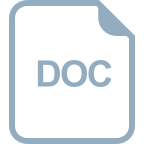
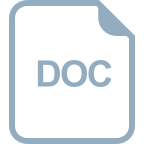
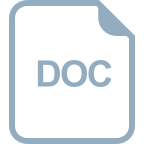
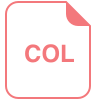
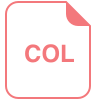
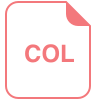
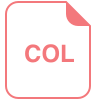
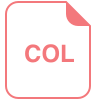
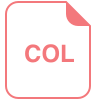

