java计算器含单选框实现进制转换
时间: 2023-10-11 10:23:48 浏览: 37
好的,我可以为您提供一个示例代码来实现这个功能。以下是Java计算器含单选框实现进制转换的示例代码:
```java
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTextField;
public class Calculator extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField input, output;
private JRadioButton binRadio, decRadio, hexRadio;
private JButton[] numButtons = new JButton[16];
private JButton addBtn, subBtn, mulBtn, divBtn, eqlBtn, clrBtn;
public Calculator() {
initUI();
}
private void initUI() {
setTitle("Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 300);
setLocationRelativeTo(null);
JPanel contentPane = new JPanel(new FlowLayout());
setContentPane(contentPane);
JPanel topPanel = new JPanel(new GridLayout(2, 1));
JPanel inputPanel = new JPanel(new FlowLayout());
JLabel label = new JLabel("Input:");
input = new JTextField(20);
inputPanel.add(label);
inputPanel.add(input);
JPanel outputPanel = new JPanel(new FlowLayout());
label = new JLabel("Output:");
output = new JTextField(20);
output.setEditable(false);
outputPanel.add(label);
outputPanel.add(output);
topPanel.add(inputPanel);
topPanel.add(outputPanel);
JPanel radioPanel = new JPanel(new FlowLayout());
binRadio = new JRadioButton("Binary");
decRadio = new JRadioButton("Decimal");
hexRadio = new JRadioButton("Hexadecimal");
ButtonGroup group = new ButtonGroup();
group.add(binRadio);
group.add(decRadio);
group.add(hexRadio);
decRadio.setSelected(true);
radioPanel.add(binRadio);
radioPanel.add(decRadio);
radioPanel.add(hexRadio);
JPanel numPanel = new JPanel(new GridLayout(4, 4));
for (int i = 0; i < 16; i++) {
numButtons[i] = new JButton(Integer.toHexString(i).toUpperCase());
numButtons[i].addActionListener(this);
numPanel.add(numButtons[i]);
}
addBtn = new JButton("+");
subBtn = new JButton("-");
mulBtn = new JButton("*");
divBtn = new JButton("/");
eqlBtn = new JButton("=");
clrBtn = new JButton("C");
addBtn.addActionListener(this);
subBtn.addActionListener(this);
mulBtn.addActionListener(this);
divBtn.addActionListener(this);
eqlBtn.addActionListener(this);
clrBtn.addActionListener(this);
numPanel.add(addBtn);
numPanel.add(subBtn);
numPanel.add(mulBtn);
numPanel.add(divBtn);
numPanel.add(eqlBtn);
numPanel.add(clrBtn);
contentPane.add(topPanel);
contentPane.add(radioPanel);
contentPane.add(numPanel);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clrBtn) {
input.setText("");
output.setText("");
return;
}
String text = ((JButton) e.getSource()).getText();
if ("+-*/".indexOf(text) >= 0) {
input.setText(input.getText() + text);
return;
}
int base = 10;
if (binRadio.isSelected()) {
base = 2;
} else if (hexRadio.isSelected()) {
base = 16;
}
try {
int value = Integer.parseInt(input.getText(), base);
output.setText(Integer.toString(value));
} catch (NumberFormatException ex) {
output.setText("Invalid input");
}
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
Calculator calc = new Calculator();
calc.setVisible(true);
});
}
}
```
这是一个简单的计算器,包含了单选框来选择进制,并且可以进行进制转换。您可以根据您的需要对代码进行修改和扩展。
相关推荐
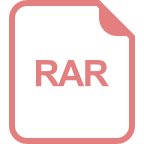














