用c语言写能监听get请求并获得URL的请求参数的函数
时间: 2024-09-20 09:11:50 浏览: 40
在 C 语言中,通常使用系统调用(如 `recv`、`parse_url` 和 `strtok` 等)来处理网络请求,特别是服务器端的 HTTP 请求。然而,这通常涉及到更底层的套接字编程和HTTP协议的理解,而不是简单的函数编写。下面是一个基础的例子,展示了如何使用 C 语言创建一个简易的命令行 GET 请求处理器,但它并不能直接监听网络连接,也没有解析 URL 参数的功能:
```c
#include <stdio.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
// 假设我们只处理 GET 请求
void handle_GET_request(char *request) {
char *url_start = strstr(request, "GET ");
if (url_start != NULL) {
url_start += strlen("GET ");
char *query_string = strchr(url_start, '?');
if (query_string != NULL) {
// query_string now points to the start of the query string
query_string++; // skip the '?' character
printf("Received parameters:\n");
while (*query_string) {
char *param_name = strtok(NULL, "&"); // split by '&' to get individual params
if (param_name == NULL) break;
char *equals_pos = strchr(param_name, '=');
if (equals_pos != NULL) {
*equals_pos++ = '\0'; // replace '=' with null terminator
printf("%s: %s\n", param_name, equals_pos); // print key-value pair
} else {
printf("%s\n", param_name); // no value found for this parameter
}
}
} else {
printf("No query parameters found.\n");
}
} else {
printf("Invalid request format.\n");
}
}
int main() {
// This is a simple example, in reality you would use a socket and listen on port
const char* request = "GET /test?param1=value1¶m2=value2 HTTP/1.1";
handle_GET_request(request);
return 0;
}
```
阅读全文
相关推荐
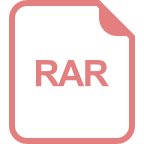
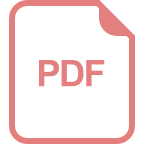
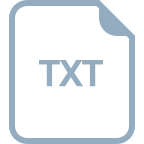
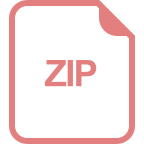
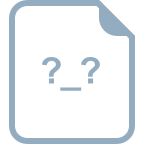
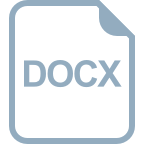