相机标定完成后,把图片转成正视图 C++
时间: 2023-10-08 11:12:52 浏览: 51
以下是使用C++和OpenCV实现相机标定并将图像转成正视图的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读入标定板图像
vector<vector<Point3f>> objectPoints;
vector<vector<Point2f>> imagePoints;
Size boardSize(9, 6);
vector<String> fileNames;
glob("calibration_images/*.jpg", fileNames);
vector<Point3f> obj;
for(int i = 0; i < boardSize.height; ++i)
for(int j = 0; j < boardSize.width; ++j)
obj.push_back(Point3f(j*0.0245, i*0.0245, 0));
for(const auto& fileName : fileNames)
{
Mat src = imread(fileName);
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
vector<Point2f> corners;
bool found = findChessboardCorners(gray, boardSize, corners);
if(found)
{
objectPoints.push_back(obj);
imagePoints.push_back(corners);
}
}
// 相机标定
Mat cameraMatrix, distCoeffs, rvec, tvec;
calibrateCamera(objectPoints, imagePoints, Size(640, 480), cameraMatrix, distCoeffs, rvec, tvec);
// 图像校正
Mat src = imread("test_image.jpg");
Mat undistorted;
undistort(src, undistorted, cameraMatrix, distCoeffs);
// 投影变换
vector<Point2f> srcPoints = {Point2f(200, 200), Point2f(600, 200), Point2f(600, 400), Point2f(200, 400)};
vector<Point2f> dstPoints = {Point2f(0, 0), Point2f(640, 0), Point2f(640, 480), Point2f(0, 480)};
Mat warpMatrix = getPerspectiveTransform(srcPoints, dstPoints);
Mat dst;
warpPerspective(undistorted, dst, warpMatrix, Size(640, 480));
// 显示结果
imshow("src", src);
imshow("undistorted", undistorted);
imshow("dst", dst);
waitKey();
return 0;
}
```
该示例代码首先读入标定板图像,并使用`findChessboardCorners()`函数检测标定板角点,然后使用`calibrateCamera()`函数对相机进行标定,得到相机矩阵和畸变系数。然后,读入测试图像,并使用`undistort()`函数对图像进行校正,去除畸变。最后,使用`getPerspectiveTransform()`函数计算投影变换矩阵,并使用`warpPerspective()`函数对图像进行投影变换,得到正视图。最后,使用`imshow()`函数显示结果,等待用户按下任意键退出程序。
相关推荐
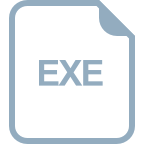
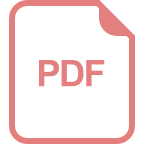














