举个使用flex和bison生成语法树共语义分析使用的例子
时间: 2024-03-05 08:49:48 浏览: 97
好的,这里举一个简单的例子。
假设我们要实现一个简单的加减乘除计算器,支持整数运算,那么我们可以先定义我们的语法规则:
```
expression: expression '+' term
| expression '-' term
| term
term: term '*' factor
| term '/' factor
| factor
factor: NUMBER
```
其中,`NUMBER` 表示整数。
接下来,我们使用 flex 生成词法分析器,使用 bison 生成语法分析器和语法树。具体实现如下:
1. 定义词法分析器(`calc.l`):
```
%{
#include "calc.tab.h"
%}
%%
[0-9]+ { yylval = atoi(yytext); return NUMBER; }
[ \t\n] ; /* skip whitespace */
[-+*/] { return yytext[0]; }
. { return yytext[0]; }
%%
int yywrap(void) {
return 1;
}
```
2. 定义语法分析器和语法树(`calc.y`):
```
%{
#include <stdio.h>
#include <stdlib.h>
int yylex(void);
void yyerror(const char *s);
int result;
%}
%union {
int val;
}
%token NUMBER
%token PLUS MINUS TIMES DIVIDE
%type <val> expression term factor
%%
expression: expression PLUS term {
$$ = $1 + $3;
}
| expression MINUS term {
$$ = $1 - $3;
}
| term {
$$ = $1;
}
term: term TIMES factor {
$$ = $1 * $3;
}
| term DIVIDE factor {
if ($3 == 0) {
yyerror("division by zero");
}
$$ = $1 / $3;
}
| factor {
$$ = $1;
}
factor: NUMBER {
$$ = $1;
}
%%
void yyerror(const char *s) {
printf("error: %s\n", s);
exit(1);
}
int main(void) {
yyparse();
printf("result: %d\n", result);
return 0;
}
```
3. 编译并运行
```
$ flex calc.l
$ bison -d calc.y
$ gcc -o calc calc.tab.c lex.yy.c
$ ./calc
```
这里我们输入类似于 `1+2*3-4/2` 的表达式,程序会自动计算出结果并输出。在这个例子中,我们使用了 flex 生成词法分析器,bison 生成语法分析器和语法树,实现了一个简单的加减乘除计算器。
相关推荐
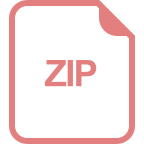














