springboot实现权限拦截的代码
时间: 2023-06-09 18:05:08 浏览: 114
好的,下面是一个基于Spring Boot实现的权限拦截的代码示例:
1. 首先,需要在pom.xml文件中添加Spring Security的依赖:
```
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
</dependencies>
```
2. 接着,在Spring Boot的配置文件application.properties中配置用户名和密码:
```
spring.security.user.name=admin
spring.security.user.password=123456
```
3. 创建一个SecurityConfig类来配置安全性。可以继承WebSecurityConfigurerAdapter类,然后覆盖configure(HttpSecurity http)方法来指定需要拦截的URL和相关权限:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}123456").roles("ADMIN")
.and()
.withUser("user").password("{noop}123456").roles("USER");
}
}
```
这段代码将/admin/** URL路径拦截并要求用户必须拥有ADMIN角色才能访问,同理,/user/** URL路径则要求用户必须拥有USER角色才能访问。其他所有URL路径都需要进行身份验证。
4. 最后,可以在控制器类中测试以下代码:
```
@RestController
public class HomeController {
@GetMapping("/")
public String home() {
return "Welcome!";
}
@GetMapping("/admin/hello")
public String adminHello() {
return "Hello Admin!";
}
@GetMapping("/user/hello")
public String userHello() {
return "Hello User!";
}
}
```
正常情况下,访问localhost:8080/会显示Welcome!,而访问localhost:8080/admin/hello则会要求用户进行认证。如果用户名和密码正确并且用户拥有ADMIN角色,则会显示Hello Admin!,否则会显示登录页面。访问localhost:8080/user/hello同理。
阅读全文
相关推荐
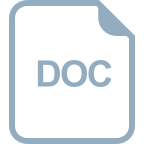
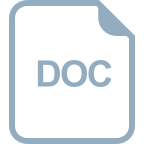
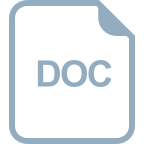


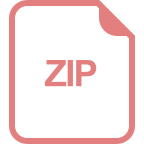
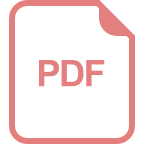
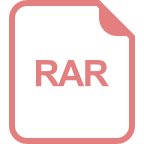
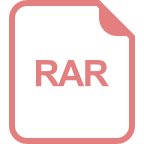
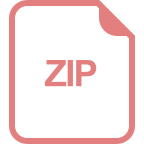
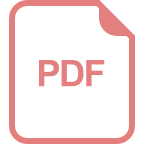
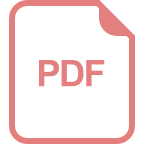
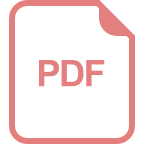
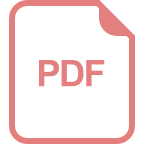
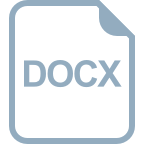
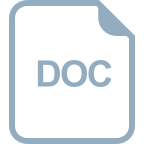


