请用脚本实现3种五子棋AI算法
时间: 2024-02-18 13:59:40 浏览: 80
为了实现三种五子棋 AI 算法,我将分别介绍极大极小值算法、Alpha-Beta 剪枝算法和蒙特卡洛树搜索算法的实现方法。以下是 Python 代码实现:
1. 极大极小值算法
```python
def minimax(board, depth, is_maximizing_player):
if depth == 0 or board.is_game_over():
return None, evaluate(board)
best_move = None
if is_maximizing_player:
max_score = -float('inf')
for move in board.get_legal_moves():
board.push(move)
_, score = minimax(board, depth - 1, False)
board.pop()
if score > max_score:
max_score = score
best_move = move
return best_move, max_score
else:
min_score = float('inf')
for move in board.get_legal_moves():
board.push(move)
_, score = minimax(board, depth - 1, True)
board.pop()
if score < min_score:
min_score = score
best_move = move
return best_move, min_score
```
2. Alpha-Beta 剪枝算法
```python
def alphabeta(board, depth, alpha, beta, is_maximizing_player):
if depth == 0 or board.is_game_over():
return None, evaluate(board)
best_move = None
if is_maximizing_player:
max_score = -float('inf')
for move in board.get_legal_moves():
board.push(move)
_, score = alphabeta(board, depth - 1, alpha, beta, False)
board.pop()
if score > max_score:
max_score = score
best_move = move
alpha = max(alpha, max_score)
if beta <= alpha:
break
return best_move, max_score
else:
min_score = float('inf')
for move in board.get_legal_moves():
board.push(move)
_, score = alphabeta(board, depth - 1, alpha, beta, True)
board.pop()
if score < min_score:
min_score = score
best_move = move
beta = min(beta, min_score)
if beta <= alpha:
break
return best_move, min_score
```
3. 蒙特卡洛树搜索算法
```python
class Node:
def __init__(self, board, move=None, parent=None):
self.board = board
self.move = move
self.parent = parent
self.wins = 0
self.visits = 0
self.children = []
def add_child(self, move, board):
node = Node(board, move, self)
self.children.append(node)
return node
def select_child(self):
return max(self.children, key=lambda c: c.wins/c.visits + sqrt(2*log(self.visits)/c.visits))
def update(self, result):
self.visits += 1
self.wins += result
def simulate(board):
current_player = board.current_player
while not board.is_game_over():
move = random.choice(board.get_legal_moves())
board.push(move)
winner = board.get_winner()
if winner == current_player:
return 1
elif winner == board.get_opponent(current_player):
return -1
else:
return 0
def monte_carlo_tree_search(board, iterations):
root_node = Node(board)
for i in range(iterations):
node = root_node
temp_board = board.copy()
while node.children:
node = node.select_child()
temp_board.push(node.move)
unvisited_moves = temp_board.get_legal_moves() - {child.move for child in node.children}
if unvisited_moves:
move = random.choice(list(unvisited_moves))
temp_board.push(move)
node = node.add_child(move, temp_board)
result = simulate(temp_board)
while node:
node.update(result)
node = node.parent
best_move = max(root_node.children, key=lambda c: c.wins/c.visits).move
return best_move
```
以上是三种不同的五子棋 AI 算法的 Python 实现,可以根据实际需求选择其中一种或多种算法进行使用。
阅读全文
相关推荐
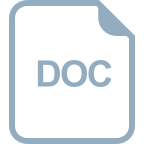
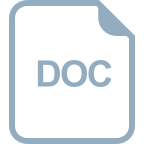
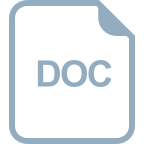
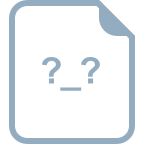
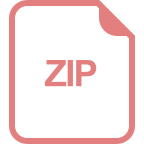
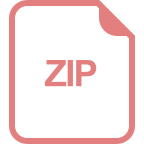
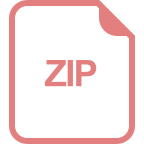
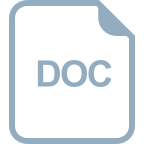
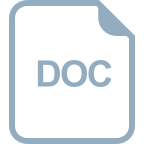
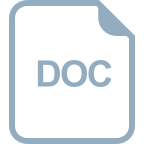
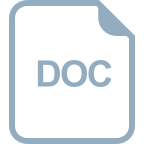
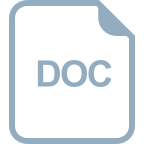
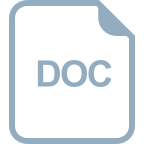
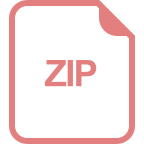
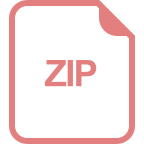
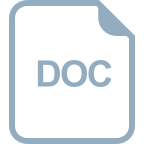
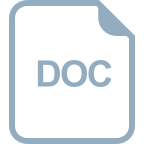
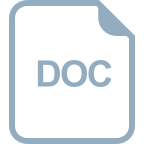
