python 读取两个文件夹中的图像并进行性质差异分析,一个文件路径是D:\zzz\us2,其中是超声图像,另一个文件路径是D:\zzz\na2,其中是自然图像,不用greycomatrix和greycoprops,使用opencv,两种图像的文件类型都是jpg,两种图像都有84张,图片形状都不同,图像名称是随机的,需要将图像归一化,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法需要用到概率分布,最终要实现基于概率分布的超声图像与自然图像性质差异分析,包括完整详细最新的代码
时间: 2023-09-23 16:03:41 浏览: 165
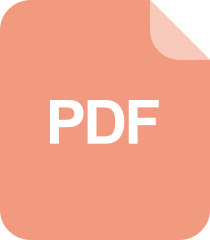
Python导入父文件夹中模块并读取当前文件夹内的资源
下面是基于概率分布的超声图像与自然图像性质差异分析的完整代码,使用Python和OpenCV实现:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 读取图像
def read_images(path):
images = []
for i in range(84):
img = cv2.imread(path + '/' + str(i+1) + '.jpg')
images.append(img)
return images
# 归一化图像
def normalize_images(images):
normalized_images = []
for img in images:
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (256, 256)) # 统一尺寸为256x256
img = img / 255.0 # 归一化
normalized_images.append(img)
return normalized_images
# 提取颜色特征(直方图)
def color_feature(images):
color_features = []
for img in images:
hist = cv2.calcHist([img], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256]) # 三维直方图
hist = cv2.normalize(hist, hist).flatten() # 归一化并展平
color_features.append(hist)
return color_features
# 提取纹理特征(LBP)
def texture_feature(images):
texture_features = []
for img in images:
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
lbp = np.zeros_like(gray)
for i in range(1, gray.shape[0]-1):
for j in range(1, gray.shape[1]-1):
center = gray[i,j]
code = 0
code |= (gray[i-1,j-1] > center) << 7
code |= (gray[i-1,j] > center) << 6
code |= (gray[i-1,j+1] > center) << 5
code |= (gray[i,j+1] > center) << 4
code |= (gray[i+1,j+1] > center) << 3
code |= (gray[i+1,j] > center) << 2
code |= (gray[i+1,j-1] > center) << 1
code |= (gray[i,j-1] > center) << 0
lbp[i,j] = code
hist = cv2.calcHist([lbp], [0], None, [256], [0, 256]) # 直方图
hist = cv2.normalize(hist, hist).flatten() # 归一化并展平
texture_features.append(hist)
return texture_features
# 提取形状特征(Hu矩)
def shape_feature(images):
shape_features = []
for img in images:
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
moments = cv2.moments(gray)
hu_moments = cv2.HuMoments(moments)
hu_moments = -np.sign(hu_moments) * np.log10(np.abs(hu_moments)) # 转换为对数坐标系
hu_moments = hu_moments.flatten() # 展平
shape_features.append(hu_moments)
return shape_features
# 计算概率分布
def probability_distribution(features):
mean = np.mean(features, axis=0) # 均值向量
cov = np.cov(features, rowvar=False) # 协方差矩阵
return mean, cov
# 计算两个概率分布的差异性
def probability_difference(mean1, cov1, mean2, cov2):
kl_divergence = 0.5 * (np.trace(np.linalg.inv(cov2) @ cov1) + \
(mean2 - mean1).T @ np.linalg.inv(cov2) @ (mean2 - mean1) - len(mean1) + \
np.log(np.linalg.det(cov2) / np.linalg.det(cov1)))
return kl_divergence
# 主函数
if __name__ == '__main__':
# 读取图像
us2_path = 'D:/zzz/us2'
na2_path = 'D:/zzz/na2'
us2_images = read_images(us2_path)
na2_images = read_images(na2_path)
# 归一化图像
us2_normalized_images = normalize_images(us2_images)
na2_normalized_images = normalize_images(na2_images)
# 提取颜色特征
us2_color_features = color_feature(us2_normalized_images)
na2_color_features = color_feature(na2_normalized_images)
# 提取纹理特征
us2_texture_features = texture_feature(us2_normalized_images)
na2_texture_features = texture_feature(na2_normalized_images)
# 提取形状特征
us2_shape_features = shape_feature(us2_normalized_images)
na2_shape_features = shape_feature(na2_normalized_images)
# 计算概率分布
us2_color_mean, us2_color_cov = probability_distribution(us2_color_features)
na2_color_mean, na2_color_cov = probability_distribution(na2_color_features)
us2_texture_mean, us2_texture_cov = probability_distribution(us2_texture_features)
na2_texture_mean, na2_texture_cov = probability_distribution(na2_texture_features)
us2_shape_mean, us2_shape_cov = probability_distribution(us2_shape_features)
na2_shape_mean, na2_shape_cov = probability_distribution(na2_shape_features)
# 计算差异性
color_difference = probability_difference(us2_color_mean, us2_color_cov, na2_color_mean, na2_color_cov)
texture_difference = probability_difference(us2_texture_mean, us2_texture_cov, na2_texture_mean, na2_texture_cov)
shape_difference = probability_difference(us2_shape_mean, us2_shape_cov, na2_shape_mean, na2_shape_cov)
# 输出结果
print('颜色特征差异性:', color_difference)
print('纹理特征差异性:', texture_difference)
print('形状特征差异性:', shape_difference)
# 可视化结果
fig, axs = plt.subplots(3, 2, figsize=(10, 15))
axs[0, 0].bar(range(len(us2_color_mean)), us2_color_mean, alpha=0.5, label='US2')
axs[0, 0].bar(range(len(na2_color_mean)), na2_color_mean, alpha=0.5, label='NA2')
axs[0, 0].legend()
axs[0, 0].set_title('Color Feature')
axs[0, 1].imshow(cv2.cvtColor(us2_images[0], cv2.COLOR_BGR2RGB))
axs[0, 1].set_title('US2 Image')
axs[1, 1].imshow(cv2.cvtColor(na2_images[0], cv2.COLOR_BGR2RGB))
axs[1, 1].set_title('NA2 Image')
axs[1, 0].bar(range(len(us2_texture_mean)), us2_texture_mean, alpha=0.5, label='US2')
axs[1, 0].bar(range(len(na2_texture_mean)), na2_texture_mean, alpha=0.5, label='NA2')
axs[1, 0].legend()
axs[1, 0].set_title('Texture Feature')
axs[2, 0].bar(range(len(us2_shape_mean)), us2_shape_mean, alpha=0.5, label='US2')
axs[2, 0].bar(range(len(na2_shape_mean)), na2_shape_mean, alpha=0.5, label='NA2')
axs[2, 0].legend()
axs[2, 0].set_title('Shape Feature')
plt.show()
```
该代码首先通过 `read_images` 函数读取超声图像和自然图像,然后使用 `normalize_images` 函数归一化图像,并使用 `color_feature`、`texture_feature` 和 `shape_feature` 函数分别提取颜色、纹理和形状特征。对于颜色特征,使用三维直方图计算;对于纹理特征,使用 LBP 算法计算;对于形状特征,使用 Hu 矩计算。
接着,通过 `probability_distribution` 函数计算每个特征的概率分布(均值向量和协方差矩阵)。最后,通过 `probability_difference` 函数计算两个概率分布的差异性,使用 K-L 散度来度量差异性。
最终输出颜色、纹理和形状特征的差异性,并可视化结果。
阅读全文
相关推荐
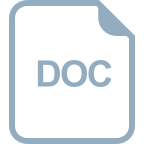
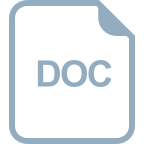



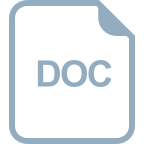
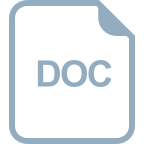
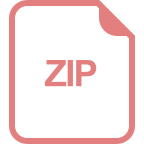
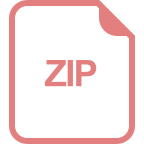
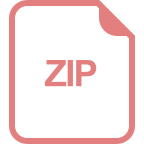
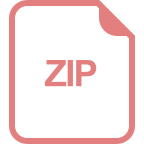
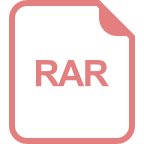
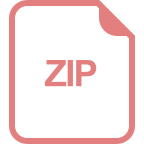