vscode通过使用 Python Django 来搭建后端接口,将训练好的模型类型为pkl加载进来,当用户上传一张水果照片时,调用接口,根据图像识别出水果种类,返回识别出的水果类型和信度值给前端具体代码实现
时间: 2024-05-05 12:19:54 浏览: 138
好的,这是一个关于如何使用Python Django搭建后端接口来识别水果图片的问题。我可以提供一些思路和代码片段供参考。
首先,需要安装Django和相关的Python库,如numpy、Pillow、joblib等。然后,创建一个Django项目和应用程序,设置数据库和路由等。
接下来,需要将训练好的模型类型为pkl加载进来,可以使用joblib库来实现。假设我们已经将模型保存在了项目目录下的`model.pkl`文件中,可以通过以下代码将其加载进来:
```python
import joblib
model = joblib.load('model.pkl')
```
然后,在应用程序中编写一个视图函数来处理用户上传的图片,并调用模型进行识别。可以使用Pillow库来打开和处理图片,使用numpy库来将图片转换为模型所需要的格式。假设我们的模型需要输入一张大小为224x224的RGB图片,可以使用以下代码来处理:
```python
from PIL import Image
import numpy as np
def predict_fruit(image):
# Open the image
img = Image.open(image)
# Resize the image to 224x224
img = img.resize((224, 224))
# Convert the image to a numpy array
img_array = np.array(img)
# Convert the image to RGB (if it is grayscale)
if len(img_array.shape) == 2:
img_array = np.stack([img_array] * 3, axis=-1)
# Convert the image to a float tensor and normalize it
img_array = img_array.astype('float32') / 255.0
# Add a batch dimension
img_array = np.expand_dims(img_array, axis=0)
# Make the prediction
pred = model.predict(img_array)
# Get the predicted class label and confidence score
class_idx = np.argmax(pred[0])
confidence = pred[0][class_idx]
# Return the predicted class label and confidence score
return class_idx, confidence
```
最后,在路由中定义一个接口,接收用户上传的图片并调用上述视图函数进行识别,返回识别结果给前端。假设我们的接口路径为`/api/predict`,可以使用以下代码来实现:
```python
from django.http import JsonResponse
def predict(request):
if request.method == 'POST' and request.FILES.get('image'):
# Get the uploaded image
image = request.FILES['image']
# Make the prediction
class_idx, confidence = predict_fruit(image)
# Convert the class index to a fruit name
fruits = ['apple', 'banana', 'orange']
fruit_name = fruits[class_idx]
# Return the prediction as JSON
return JsonResponse({'fruit': fruit_name, 'confidence': float(confidence)})
else:
return JsonResponse({'error': 'Invalid request'})
```
以上是一个简单的使用Python Django搭建后端接口来识别水果图片的示例。当用户上传一张水果照片时,调用接口,根据图像识别出水果种类,返回识别出的水果类型和信度值给前端。
阅读全文
相关推荐
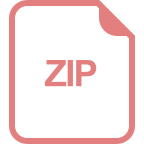
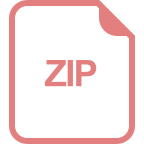
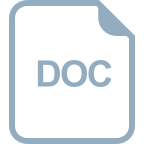
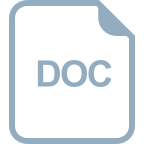
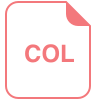
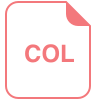
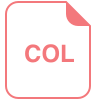
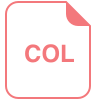
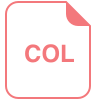
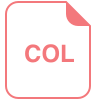
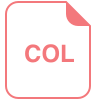
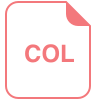
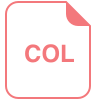
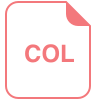
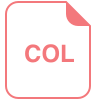
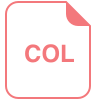