前向迭代器代码示例C++的
时间: 2023-11-14 11:11:02 浏览: 63
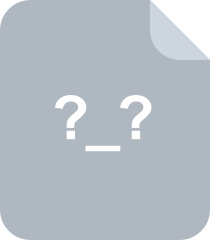
迭代算法的c++代码版
下面是一个简单的前向迭代器代码示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 定义一个前向迭代器类
class MyIterator {
public:
// 构造函数
MyIterator(vector<int>::iterator it) : _it(it) {}
// 重载解引用操作符
int& operator*() {
return *_it;
}
// 重载 ++ 操作符
MyIterator& operator++() {
++_it;
return *this;
}
// 重载 == 操作符
bool operator==(const MyIterator& other) const {
return _it == other._it;
}
// 重载 != 操作符
bool operator!=(const MyIterator& other) const {
return !(*this == other);
}
private:
vector<int>::iterator _it; // 存储迭代器位置
};
int main() {
// 创建一个 vector 容器
vector<int> vec = {1, 2, 3, 4, 5};
// 使用自定义的迭代器遍历 vector 容器
for (MyIterator it = vec.begin(); it != vec.end(); ++it) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
这个代码示例中,我们定义了一个 `MyIterator` 类,它包含一个指向 `vector<int>` 的迭代器 `_it`。我们重载了解引用操作符 `*`,使得 `MyIterator` 类的对象可以像指针一样解引用。同时,我们也重载了 `++` 操作符,使得迭代器可以像指针一样递增。最后,我们还重载了 `==` 和 `!=` 操作符,以便在遍历时判断迭代器是否到达了容器的末尾。在 `main` 函数中,我们使用自定义的迭代器遍历了一个 vector 容器,并输出了容器中的元素。
阅读全文
相关推荐
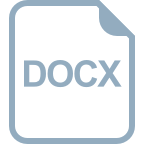
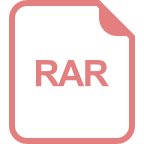
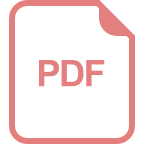
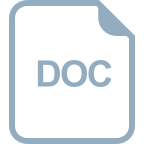
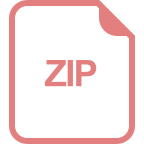
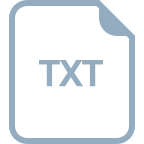
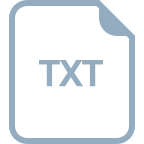
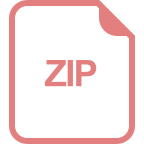
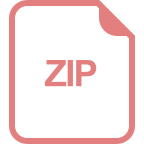
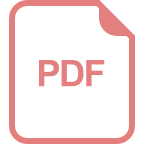
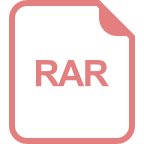
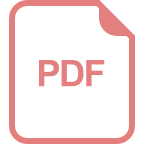
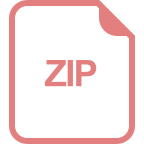
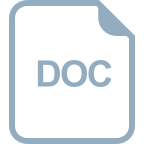
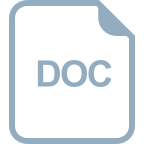