C语言字符串拼接后存入一个新的数组
时间: 2024-03-18 22:40:53 浏览: 22
在C语言中,可以使用sprintf函数将两个字符串拼接并存入一个新的数组中。sprintf函数的第一个参数是目标字符串,第二个参数是格式化字符串,其中可以使用%s表示要拼接的字符串,例如:
```
char str1[50] = "Hello";
char str2[20] = "world!";
char result[70];
sprintf(result, "%s %s", str1, str2);
```
执行该代码后,result的值将变为"Hello world!"。需要注意的是,要确保目标数组的长度足够容纳拼接后的字符串,否则会导致缓冲区溢出的问题。
相关问题
C语言将一串字符串拼接为16进制数组
以下是将字符串拼接为16进制数组的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
int len = strlen(str);
unsigned char* hex = (unsigned char*)malloc(len * 2 * sizeof(unsigned char));
for (int i = 0; i < len; i++) {
sprintf((char*)(hex + (i * 2)), "%02X", str[i]);
}
for (int i = 0; i < len * 2; i++) {
printf("%c ", *(hex + i));
}
free(hex);
return 0;
}
```
上述代码中,我们首先定义了一个字符串 `str`,然后计算字符串长度并分配了一个大小为 `len * 2` 的 unsigned char 数组 `hex`,用于存储16进制结果。然后,我们使用 `sprintf` 函数将字符转换为16进制形式,并将结果存储在 `hex` 数组中。最后,我们遍历 `hex` 数组并打印出其内容。
注意,此代码示例假设输入的字符串中没有空字符,如果有空字符,则需要相应地调整代码。
C语言将一串字符串拼接为uint8_t数组
可以使用C语言中的字符串操作函数和位运算来拼接字符串为uint8_t数组。
首先需要声明一个uint8_t类型的数组和一个指向char类型的字符串的指针。
```c
uint8_t buffer[1024];
char *str = "hello world";
```
然后使用strlen函数获取字符串的长度,遍历字符串并将每个字符转换为uint8_t类型并存储到数组中。可以使用位运算将两个uint8_t类型合并为一个。
```c
size_t len = strlen(str);
for (int i = 0; i < len; i++) {
uint8_t c = str[i];
buffer[i/2] |= (i%2==0) ? c<<4 : c;
}
```
最后,我们得到的buffer数组即为拼接后的uint8_t数组。
相关推荐
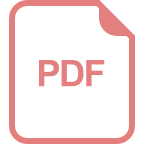
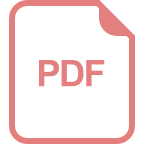
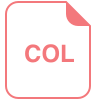
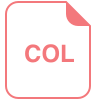
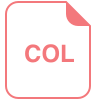
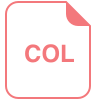
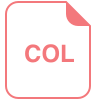







