C语言 如何数据写入文件
时间: 2024-05-05 08:16:14 浏览: 71
在C语言中,可以使用文件操作函数将数据写入文件。下面是一个简单的示例:
```c
#include <stdio.h>
int main() {
FILE *fp;
char str[] = "Hello, world!";
// 打开文件
fp = fopen("file.txt", "w");
// 写入数据
fputs(str, fp);
// 关闭文件
fclose(fp);
return 0;
}
```
以上代码中,使用`fopen`函数打开名为`file.txt`的文件,并指定以写入模式打开。然后使用`fputs`函数将字符串`"Hello, world!"`写入文件。最后使用`fclose`函数关闭文件。
除了`fputs`函数,还可以使用其他文件写入函数,例如`fprintf`函数和`fwrite`函数等。具体使用哪个函数取决于需要写入的数据类型和格式。
相关问题
c语言结构体写入文件
以下是使用C语言结构体写入文件的示例代码:
```c
#include <stdio.h>
// 定义结构体
typedef struct {
int stu_id;
char name[20];
} Stu;
int main() {
// 定义一个文件指针
FILE *fp;
// 初始化结构体数组
Stu stuw[2] = { {20180001, "xiaoming" }, {20180002, "小明"} };
// 打开文件,没有文件自动创建
fp = fopen("student.dat","wb"); // b:表示以二进制写入
// 写入数据
fwrite((char*)stuw, sizeof(Stu), 2, fp); // 2:表示将数组中两个元素写入文件
// 关闭文件
fclose(fp);
return 0;
}
```
C语言结构体写入文件
在C语言中,结构体(struct)是一种用户自定义的数据类型,用于组合不同类型的变量。要将结构体写入文件,通常需要使用文件操作函数,如`fopen`、`fwrite`或`fprintf`。以下是一个简单的步骤:
1. 定义结构体:
```c
typedef struct {
int id;
float value;
char name[20];
} MyStruct;
```
2. 创建结构体实例:
```c
MyStruct myObj;
myObj.id = 1;
myObj.value = 3.14;
strcpy(myObj.name, "Example");
```
3. 打开文件以写入:
```c
FILE* file = fopen("output.txt", "w");
if (file == NULL) {
perror("Error opening file");
return 1;
}
```
4. 写入结构体到文件:
```c
// 使用fwrite写入结构体
size_t written = fwrite(&myObj, sizeof(MyStruct), 1, file);
if (written != 1) {
perror("Error writing to file");
fclose(file);
return 1;
}
```
或者使用`fprintf`格式化输出:
```c
fprintf(file, "%d %f %s\n", myObj.id, myObj.value, myObj.name);
```
记得在结束写入后关闭文件:
```c
fclose(file);
```
阅读全文
相关推荐
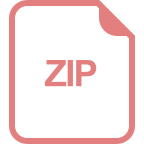
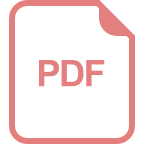
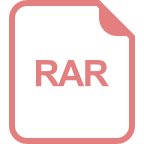













