$.ajax({ url: editor.config.imageUploadURL, type: 'POST', data: formData, processData: false, contentType: false, success: function(data, textStatus, jqXHR){ var isNew; if (jqXHR.status == 200) { maxWidth = Math.min(inputWidth, 700); maxHeight = Math.min(inputHeight, 700); width = inputWidth; height = inputHeight; if (inputWidth > maxWidth){ width = maxWidth; height = inputHeight * maxWidth / inputWidth; } if (height > maxHeight){ width = width * maxHeight / inputHeight; height = maxHeight; } var html = '<img src="' + data + '" class="image-editor draggable" data-width="' + inputWidth + '" data-height="' + inputHeight + '" width="' + width + '">'; $("#" + curr).append(html); } },为图片添加拖拽功能,并且页面刷新之后也能拖拽
时间: 2024-01-07 08:04:20 浏览: 75
要为图片添加拖拽功能,可以使用jQuery UI库中的draggable方法。可以在成功上传图片后,动态添加类名为"draggable"的img标签,并在页面刷新时重新初始化。
代码示例:
1. 引入jQuery和jQuery UI库
```html
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
```
2. 修改上传成功后的回调函数,添加拖拽功能
```javascript
success: function(data, textStatus, jqXHR){
// 省略部分代码
var html = '<img src="' + data + '" class="image-editor draggable" data-width="' + inputWidth + '" data-height="' + inputHeight + '" width="' + width + '">';
$("#" + curr).append(html);
// 重新初始化拖拽功能
$(".draggable").draggable({
containment: "parent"
});
}
```
其中,containment选项指定拖拽范围为父元素,即图片只能在其父元素内拖拽。
相关问题
$.ajax发送contentType: 'multipart/form-data"',
`$.ajax()` 方法可以用来发送异步 HTTP 请求。当我们需要上传文件时,需要将 `contentType` 设置为 `'multipart/form-data'`,这样浏览器就会以多部分的形式发送数据,包括文件和其他表单数据。例如:
```javascript
$.ajax({
url: '/upload',
type: 'POST',
data: formData,
contentType: false,
processData: false,
success: function(response) {
console.log('上传成功');
},
error: function(error) {
console.log('上传失败');
}
});
```
在上面的示例中,`data` 是一个 `FormData` 对象,它包含了要上传的文件和其他表单数据。`contentType` 被设置为 `false`,因为我们使用的是 `FormData` 对象,浏览器会自动设置正确的 `Content-Type`。`processData` 也被设置为 `false`,因为我们不需要将数据转换为字符串。在成功或失败的回调函数中,我们可以处理上传结果。
$.ajax form-data
$.ajax是一个jQuery中的方法,用于进行异步的HTTP请求。它可以通过发送不同类型的数据到服务器来实现与后端进行交互。
当你使用$.ajax发送表单数据时,你可以使用“form-data”作为请求的数据类型。这意味着你可以通过将表单数据序列化为字符串并将其发送到服务器来提交表单。
以下是一个使用$.ajax发送表单数据的示例代码:
```javascript
// 获取表单数据
var formData = $('#myForm').serialize();
$.ajax({
url: 'your-url', // 服务器端接口地址
type: 'POST', // 请求方式
data: formData, // 表单数据
processData: false, // 禁止自动处理数据
contentType: false, // 禁止自动设置请求头
success: function(response) {
// 请求成功后的回调函数
console.log(response);
},
error: function(xhr, status, error) {
// 请求失败后的回调函数
console.log(error);
}
});
```
在这个例子中,你需要替换'your-url'为你的服务器端接口地址,并确保你在HTML中有一个id为'myForm'的表单。该代码将会获取表单数据并将其作为'form-data'发送到服务器端。
请注意,我们设置了'processData'和'contentType'的值为false,这是因为我们希望手动处理表单数据而不是让jQuery自动处理。这也确保了正确的请求头被设置为'multipart/form-data'。
希望这个回答对你有所帮助!如有更多问题,请随时提问。
阅读全文
相关推荐
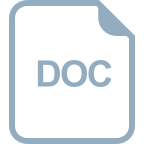
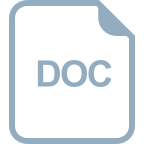
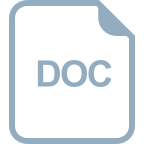
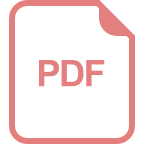
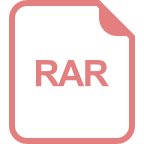
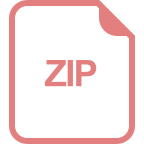
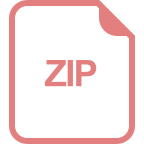
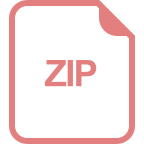
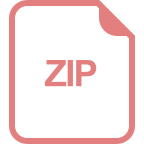
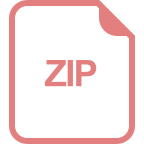





