根据行数列数左上角右上角右下角算出矩阵所有坐标及间距,要求C Shap语言简单封装
时间: 2023-05-31 11:03:58 浏览: 89
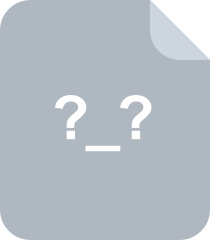
用c语言实现矩阵的运算

以下是一个简单的C#语言封装示例,可以根据行数、列数、左上角坐标、右上角坐标和右下角坐标计算出矩阵中所有点的坐标和间距:
```
public class MatrixCalculator
{
private int rows;
private int cols;
private Point topLeft;
private Point topRight;
private Point bottomRight;
public MatrixCalculator(int rows, int cols, Point topLeft, Point topRight, Point bottomRight)
{
this.rows = rows;
this.cols = cols;
this.topLeft = topLeft;
this.topRight = topRight;
this.bottomRight = bottomRight;
}
public List<Point> GetAllPoints()
{
List<Point> points = new List<Point>();
// Calculate the x and y distance between points
double xDistance = (topRight.X - topLeft.X) / (cols - 1);
double yDistance = (bottomRight.Y - topLeft.Y) / (rows - 1);
// Loop through all rows and columns to calculate each point
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
double x = topLeft.X + xDistance * j;
double y = topLeft.Y + yDistance * i;
points.Add(new Point(x, y));
}
}
return points;
}
}
```
使用示例:
```
// Define the matrix parameters
int rows = 3;
int cols = 4;
Point topLeft = new Point(0, 0);
Point topRight = new Point(10, 0);
Point bottomRight = new Point(10, 5);
// Create a new matrix calculator instance
MatrixCalculator calculator = new MatrixCalculator(rows, cols, topLeft, topRight, bottomRight);
// Get all points in the matrix
List<Point> points = calculator.GetAllPoints();
// Print out the coordinates of each point
foreach (Point point in points)
{
Console.WriteLine("({0}, {1})", point.X, point.Y);
}
```
阅读全文
相关推荐
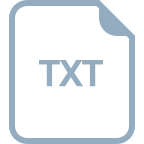


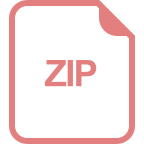
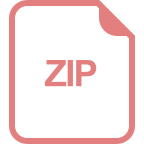
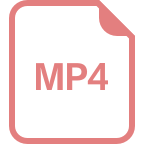
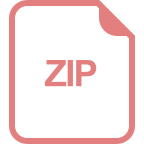
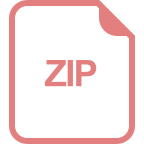
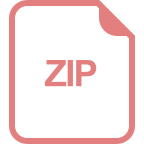
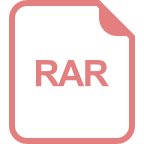
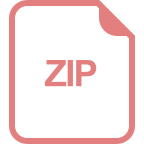