c = sorted(cnts, key=cv2.contourArea, reverse=True)[0]
时间: 2024-05-22 15:17:16 浏览: 14
这段代码是什么意思?
这段代码是对一个轮廓列表 cnts 进行按照面积大小排序,然后取出面积最大的轮廓 c,并将其赋值给变量 c。具体是通过调用 OpenCV 库中的 cv2.contourArea 函数对轮廓进行计算得到轮廓的面积,然后使用 sorted 函数进行排序,最后按照降序排列(即面积最大的轮廓在首位)并取出第一个元素。
相关问题
c = sorted(cnts, key=cv2.contourArea, reverse=True)[0]报错
这个错误可能有多种原因,需要查看完整的代码和错误信息才能确定准确的原因。但是,从这段代码来看,可能出现以下几种情况:
1. "cnts" 可能为空,导致 "sorted" 函数无法进行排序。此时可以添加一个判断,如下所示:
```
if len(cnts) > 0:
c = sorted(cnts, key=cv2.contourArea, reverse=True)[0]
```
2. "sorted" 函数中 "key" 参数的使用可能不正确。在这个例子中,我们使用 "cv2.contourArea" 函数来计算轮廓的面积,并将其作为排序依据。但如果 "cv2.contourArea" 函数的使用不正确,也会导致错误。可以检查一下 "cv2.contourArea" 函数的使用是否正确。
3. "reverse" 参数的使用也可能不正确。在这个例子中,我们将 "reverse" 参数设置为 "True",表示按照轮廓面积降序排序。但如果 "reverse" 参数设置不正确,也可能导致错误。
4. 可能还有其他未知的原因导致错误。需要查看完整的代码和错误信息才能确定准确的原因。
c = sorted(cnts, key=cv2.contourArea, reverse=True)[0】
这段代码的作用是对 `cnts` 中的所有轮廓按照面积从大到小排序,并将面积最大的轮廓赋值给变量 `c`。
具体来说,`sorted()` 函数是 Python 内置的排序函数,它的第一个参数 `cnts` 是要排序的对象,第二个参数 `key` 是指定排序依据的函数,这里使用 `cv2.contourArea` 函数来计算轮廓的面积。第三个参数 `reverse` 是一个布尔值,表示是否降序排列。由于我们希望将轮廓按照面积从大到小排列,所以 `reverse` 被设置为 `True`。
最后,使用索引 `[0]` 取出排序后面积最大的轮廓,并将其赋值给变量 `c`。
相关推荐
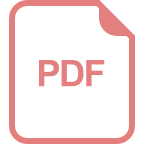
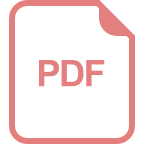
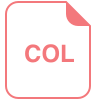
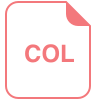
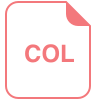
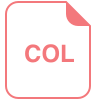
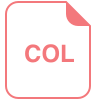







