用C++ 编写程序对10个整数进行排序。随机输入10个整数,然后进行排序,再输出从小到大的顺序和从大到小的顺序。
时间: 2024-09-20 12:10:12 浏览: 44
在C++中,你可以使用`<algorithm>`库提供的排序算法如`sort()`来实现这个功能。这里是一个简单的例子,使用冒泡排序算法和快速排序算法分别实现从大到小和从小到大的排序:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib> // 需要头文件用于获取随机数
#include <ctime> // 时间函数
// 冒泡排序从小到大
void bubbleSort(std::vector<int>& nums) {
int n = nums.size();
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (nums[j] > nums[j + 1]) {
std::swap(nums[j], nums[j + 1]);
}
}
}
}
// 快速排序从大到小
void quickSort(std::vector<int>& nums, int low, int high) {
if (low < high) {
int pivot = nums[high]; // 最后一个元素作为基准
int i = low - 1;
for (int j = low; j < high; ++j) {
if (nums[j] >= pivot) {
i++;
std::swap(nums[i], nums[j]);
}
}
std::swap(nums[i + 1], nums[high]);
quickSort(nums, low, i);
quickSort(nums, i + 2, high);
}
}
int main() {
srand(time(0)); // 设置随机种子
std::vector<int> nums(10); // 创建一个包含10个整数的向量
// 随机生成10个整数并填充向量
for (auto& num : nums) {
num = rand() % 100; // 取0-99之间的随机整数
}
// 输出原始数组
std::cout << "Original array: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << "\n";
// 冒泡排序从小到大
bubbleSort(nums);
std::cout << "Sorted in ascending order: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << "\n";
// 快速排序从大到小
quickSort(nums, 0, nums.size() - 1);
std::cout << "Sorted in descending order: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << "\n";
return 0;
}
```
阅读全文
相关推荐
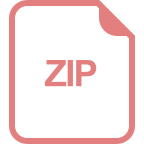















