请详细解释下这段代码Rect<float> Framer::ComputeActiveCropRegion(int frame_number) { const float min_crop_size = 1.0f / options_.max_zoom_ratio; const float new_x_crop_size = std::clamp(region_of_interest_.width * options_.target_crop_to_roi_ratio, min_crop_size, 1.0f); const float new_y_crop_size = std::clamp(region_of_interest_.height * options_.target_crop_to_roi_ratio, min_crop_size, 1.0f); // We expand the raw crop region to match the desired output aspect ratio. const float target_aspect_ratio = static_cast<float>(options_.input_size.height) / static_cast<float>(options_.input_size.width) * static_cast<float>(options_.target_aspect_ratio_x) / static_cast<float>(options_.target_aspect_ratio_y); Rect<float> new_crop; if (new_x_crop_size <= new_y_crop_size * target_aspect_ratio) { new_crop.width = std::min(new_y_crop_size * target_aspect_ratio, 1.0f); new_crop.height = new_crop.width / target_aspect_ratio; } else { new_crop.height = std::min(new_x_crop_size / target_aspect_ratio, 1.0f); new_crop.width = new_crop.height * target_aspect_ratio; } const float roi_x_mid = region_of_interest_.left + (region_of_interest_.width / 2); const float roi_y_mid = region_of_interest_.top + (region_of_interest_.height / 2); new_crop.left = std::clamp(roi_x_mid - (new_crop.width / 2), 0.0f, 1.0f - new_crop.width); new_crop.top = std::clamp(roi_y_mid - (new_crop.height / 2), 0.0f, 1.0f - new_crop.height); const float normalized_crop_strength = std::powf(options_.crop_filter_strength, ElapsedTimeMs(timestamp_) / kUnitTimeSlice); active_crop_region_.left = IirFilter(active_crop_region_.left, new_crop.left, normalized_crop_strength); active_crop_region_.top = IirFilter(active_crop_region_.top, new_crop.top, normalized_crop_strength); active_crop_region_.width = IirFilter( active_crop_region_.width, new_crop.width, normalized_crop_strength); active_crop_region_.height = IirFilter( active_crop_region_.height, new_crop.height, normalized_crop_strength); timestamp_ = base::TimeTicks::Now(); if (VLOG_IS_ON(2)) { DVLOGFID(2, frame_number) << "region_of_interest=" << region_of_interest_; DVLOGFID(2, frame_number) << "new_crop_region=" << new_crop; DVLOGFID(2, frame_number) << "active_crop_region=" << active_crop_region_; } return active_crop_region_; }
时间: 2023-09-20 12:07:15 浏览: 45
这段代码是一个函数,函数名为ComputeActiveCropRegion,它接收一个整数frame_number作为参数,并返回一个Rect<float>类型的对象active_crop_region_。该函数的目的是计算当前帧的裁剪区域,并将其存储在active_crop_region_中,以便后续使用。
函数的实现过程如下:
首先,函数定义了一个常量min_crop_size,它表示最小的裁剪尺寸,即当裁剪区域的宽度或高度小于等于该值时,裁剪区域将不再缩小。然后,函数根据region_of_interest_的宽度和高度计算出新的裁剪区域的宽度和高度,这里使用了std::clamp函数将宽度和高度限制在[min_crop_size, 1.0f]的范围内。
接着,函数计算出目标宽高比target_aspect_ratio,该值是根据输入图像的尺寸和目标宽高比计算而来的。然后,函数根据新的裁剪区域的宽度和高度以及目标宽高比,计算出新的裁剪区域的左上角坐标和宽高。
最后,函数使用IIR滤波器将新的裁剪区域的左上角坐标和宽高与之前计算得到的active_crop_region_进行平滑处理,并将结果存储在active_crop_region_中。同时,函数还更新了timestamp_的值,用于计算IIR滤波器的时间间隔。
如果开启了VLOG(即日志调试),函数还会输出region_of_interest_、new_crop_region和active_crop_region_等信息。
总的来说,该函数的作用是计算当前帧的裁剪区域并将其平滑处理,以便后续使用。
相关问题
把这段代码从c++转换为c代码Rect<float> Framer::ComputeActiveCropRegion(int frame_number) { const float min_crop_size = 1.0f / options_.max_zoom_ratio; const float new_x_crop_size = std::clamp(region_of_interest_.width * options_.target_crop_to_roi_ratio,
把这段 C++ 代码转换成 C 代码并不是一件简单的事情,因为 C++ 和 C 有许多语法上的不同,C++ 有许多 C 中没有的特性,例如模板、类和命名空间等等。因此,我们需要对代码进行适当的修改和调整。以下是将 C++ 代码转换为 C 代码的一种可能的方式:
```c
struct Rect {
float x, y, width, height;
};
struct FramerOptions {
float max_zoom_ratio;
float target_crop_to_roi_ratio;
};
struct Framer {
struct Rect region_of_interest_;
struct FramerOptions options_;
};
float clamp(float value, float min_value, float max_value) {
if (value < min_value) {
return min_value;
} else if (value > max_value) {
return max_value;
} else {
return value;
}
}
struct Rect ComputeActiveCropRegion(struct Framer *framer, int frame_number) {
const float min_crop_size = 1.0f / framer->options_.max_zoom_ratio;
const float new_x_crop_size = clamp(framer->region_of_interest_.width * framer->options_.target_crop_to_roi_ratio, min_crop_size, 1.0f);
struct Rect active_crop_region;
active_crop_region.x = framer->region_of_interest_.x + (framer->region_of_interest_.width - new_x_crop_size) / 2.0f;
active_crop_region.y = framer->region_of_interest_.y;
active_crop_region.width = new_x_crop_size;
active_crop_region.height = framer->region_of_interest_.height;
return active_crop_region;
}
```
需要注意的是,C 中没有命名空间的概念,因此我们将结构体 `Rect`、`FramerOptions` 和 `Framer` 放在了全局命名空间中。另外,C 中没有 `std::clamp` 函数,我们需要手动实现一个 `clamp` 函数来实现相同的功能。最后,建议根据实际情况对代码进行适当的调整,以便更好地适应 C 语言的语法和特性。
请详细解释下这段代码void Framer::OnNewFaceRegions(int frame_number, const std::vector<Rect<float>>& faces) { if (faces.empty()) { // TODO(jcliang): See if we want to zoom out to whole frame. return; } float min_x0 = 1.0f,
这段代码是一个函数的实现,函数名为Framer::OnNewFaceRegions。该函数的作用是处理输入的人脸检测结果。函数的参数包括一个整数frame_number,表示当前处理的帧号,以及一个表示人脸位置的向量faces。
函数首先进行一个判断,判断输入的人脸位置向量faces是否为空。如果为空,则返回函数,不做任何处理。
如果人脸位置向量faces不为空,则函数会执行下面的代码块。该代码块的作用是计算人脸位置向量faces中所有人脸位置的最小x0值和最大x1值。这里的x0和x1分别表示人脸位置矩形的左上角x坐标和右下角x坐标。代码块中定义了两个变量min_x0和max_x1,它们的初值分别为1.0f和0.0f,表示最小值和最大值的初始范围。然后,代码块使用一个循环遍历人脸位置向量faces中的所有人脸位置矩形,计算每个人脸位置矩形的x0和x1值,并将它们与min_x0和max_x1进行比较,更新最小值和最大值。最后,代码块结束并返回函数。
该代码主要用于计算人脸位置矩形的最小x0值和最大x1值,以便在后续的处理中使用。在实际应用中,这些值可以用于确定需要处理的图像区域,以提高处理速度和精度。
相关推荐
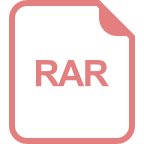












