windlx求矩阵相乘
时间: 2023-11-24 22:07:27 浏览: 83
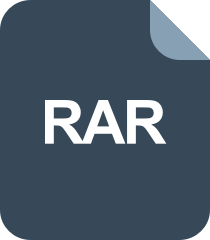
juzhencheng.rar_windlx_汇编 矩阵_汇编矩阵
矩阵相乘的原理是:若 $A$ 是 $m$ 行 $n$ 列的矩阵,$B$ 是 $n$ 行 $p$ 列的矩阵,那么它们的乘积 $C=AB$ 是一个 $m$ 行 $p$ 列的矩阵,其中 $C$ 的第 $i$ 行第 $j$ 列的元素可以表示为:
$$
C_{i,j}=\sum_{k=1}^{n}A_{i,k}B_{k,j}
$$
以下是矩阵相乘的 Python 代码实现:
```python
def matrix_multiply(A, B):
m = len(A)
n = len(A[0])
p = len(B[0])
C = [[0] * p for _ in range(m)]
for i in range(m):
for j in range(p):
for k in range(n):
C[i][j] += A[i][k] * B[k][j]
return C
```
其中,$A$ 和 $B$ 分别为两个要相乘的矩阵,返回的 $C$ 为相乘后的结果矩阵。
阅读全文
相关推荐
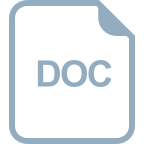
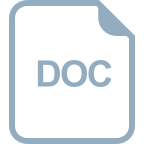

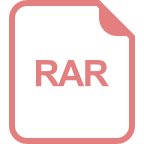



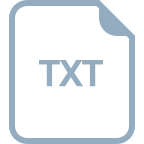
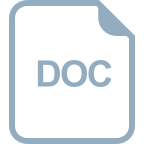
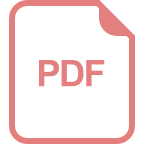
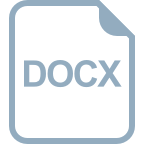
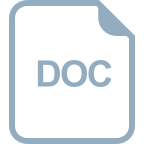
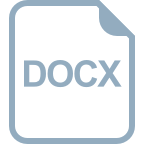
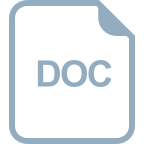
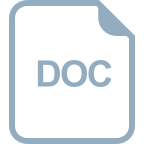
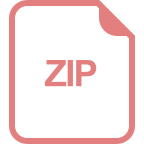