用c++编写一个万年历
时间: 2023-09-06 22:04:30 浏览: 142
### 回答1:
好的,以下是用C语言编写的一个简单万年历程序:
```c
#include <stdio.h>
int days_of_month[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
char week[7][4] = {"日", "一", "二", "三", "四", "五", "六"};
int is_leap_year(int year) {
return ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0));
}
int get_days_of_month(int year, int month) {
if (month == 2 && is_leap_year(year)) {
return 29;
} else {
return days_of_month[month];
}
}
int main() {
int year, month;
printf("请输入年份: ");
scanf("%d", &year);
printf("请输入月份: ");
scanf("%d", &month);
printf("====================\n");
printf(" %d 年 %d 月\n", year, month);
printf("====================\n");
printf("日 一 二 三 四 五 六\n");
// 获得当月1号是星期几
int first_day_of_month = 1;
for (int i = 1900; i < year; i++) {
first_day_of_month += (is_leap_year(i) ? 366 : 365);
}
for (int i = 1; i < month; i++) {
first_day_of_month += get_days_of_month(year, i);
}
first_day_of_month = first_day_of_month % 7;
for (int i = 0; i < first_day_of_month; i++) {
printf(" ");
}
// 输出当月所有天数
int days_of_current_month = get_days_of_month(year, month);
for (int i = 1; i <= days_of_current_month; i++) {
printf("%2d ", i);
if ((first_day_of_month + i) % 7 == 0) {
printf("\n");
}
}
if ((first_day_of_month + days_of_current_month) % 7 != 0) {
printf("\n");
}
printf("====================\n");
return 0;
}
```
此程序可以将输入的年份和月份作为参数,输出指定年月的日历,例如:
```
请输入年份: 2022
请输入月份: 11
====================
2022 年 11 月
====================
日 一 二 三 四 五 六
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30
====================
```
### 回答2:
编写一个万年历的C程序涉及到以下几个步骤:
1. 定义函数来判断某一年是否为闰年。闰年的判断规则是:
- 如果该年份能被4整除但不能被100整除,则是闰年。
- 如果该年份能被400整除,则也是闰年。
2. 定义函数来计算某一年1月1日是星期几。我们可以利用Zeller公式来计算,该公式的逻辑是:
- 根据公式: h = (q + [(13 * (m + 1)) / 5] + K + [K / 4] + [J / 4] - 2 * J) mod 7
- q 是日期,m 是月份(3代表3月,4代表4月,...,在公式中1月和2月被视为前一年的13月和14月),y 是年份的前两位数字,K 是年份的后两位数字,J 是年份的前两位数字。
- h 的值表示当年1月1日的星期几,0代表星期日,1代表星期一,以此类推。
3. 在主函数中,首先获取用户输入的年份,并使用闰年判断函数来确定该年份是否为闰年。然后使用星期计算函数来计算该年份的1月1日是星期几。
4. 根据1月1日的星期几,构建一个二维数组来表示整个年份的日历。数组的行表示星期,从0到6对应于星期日到星期六,列表示日期的排列。
- 我们可以使用循环来填充数组,首先填充1月,然后是2月,以此类推,直到12月。在填充的过程中,需要根据每个月的天数以及1月1日是星期几来确定日期的排列。
5. 最后,打印输出整个年份的万年历。
注意:以上步骤只是程序的基本框架,具体的实现细节还需根据个人的编程能力和经验来完善。
### 回答3:
编写一个万年历的程序需要考虑到年、月、日的变化和计算规则。以C语言为例,可以使用基本的控制流和算术运算来实现。以下是一个简单的实现:
```
#include <stdio.h>
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
return 1; // 是闰年
} else {
return 0; // 不是闰年
}
}
int main() {
int month, year;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
int daysInMonth = 31;
if (month == 2) {
if (isLeapYear(year)) {
daysInMonth = 29;
} else {
daysInMonth = 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
daysInMonth = 30;
}
printf("\n------------------------\n");
printf(" %d年%d月\n", year, month);
printf("------------------------\n");
printf("日 一 二 三 四 五 六\n");
int dayOfWeek = 0; // 0表示星期日
// 计算并打印每天的日期
for (int day = 1; day <= daysInMonth; day++) {
if (day == 1) {
// 根据1号是星期几,确定第一行空格的数量
for (int i = 0; i < dayOfWeek; i++) {
printf(" ");
}
}
printf("%2d ", day);
// 每7天换行
if ((dayOfWeek + day) % 7 == 6) {
printf("\n");
}
dayOfWeek = (dayOfWeek + 1) % 7;
}
printf("\n------------------------\n");
return 0;
}
```
这个程序首先判断输入的年份是否为闰年,然后根据月份确定该月的天数。接着,使用一个循环打印出该月的日历,根据每个月的1号是星期几对齐打印内容。最后,根据每7天换行。这样可以得到一个简单的万年历。
注:以上代码只是一个范例,可能没有考虑一些特殊情况,如输入的年份和月份是否合法等。实际使用中可以根据自己的需求和补充完善相关功能。
阅读全文
相关推荐

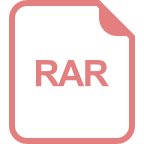














