pyqt5入门教程 csdn azuremouse
时间: 2023-06-18 07:08:57 浏览: 63
好的,以下是 PyQT5 入门教程,由 CSDN 的 azuremouse 提供。
PyQt5 是基于 Python 语言和 Qt 库的一款 GUI 工具包,可以用于创建桌面应用程序。本教程将介绍 PyQt5 的基础知识和使用方法。
## 1. 安装 PyQt5
在使用 PyQt5 之前,需要先安装 PyQt5 库。可以通过 pip 命令进行安装:
```
pip install PyQt5
```
## 2. 创建窗口
使用 PyQt5 创建窗口需要用到 `QtWidgets` 模块中的 `QApplication` 和 `QMainWindow` 类。`QApplication` 类用于创建应用程序,`QMainWindow` 类用于创建主窗口。
以下是一个简单的 PyQt5 窗口程序:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 窗口程序")
self.setGeometry(100, 100, 400, 300)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,首先导入了 `QApplication` 和 `QMainWindow` 类。然后定义了一个 `MainWindow` 类,继承自 `QMainWindow` 类,用于创建主窗口。在 `MainWindow` 类的构造函数中,设置了窗口标题和大小。最后在 `__main__` 函数中创建了一个 `QApplication` 对象和一个 `MainWindow` 对象,并显示窗口。
## 3. 添加控件
在 PyQt5 中,可以使用各种控件来实现各种功能。常用的控件包括标签(`QLabel`)、按钮(`QPushButton`)、文本框(`QLineEdit`)等。可以使用 `addWidget` 方法将控件添加到窗口中。
以下是一个添加标签和按钮的 PyQt5 窗口程序:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 窗口程序")
self.setGeometry(100, 100, 400, 300)
# 添加标签
label = QLabel('Hello PyQt5', self)
label.move(150, 50)
# 添加按钮
button = QPushButton('退出', self)
button.move(150, 100)
button.clicked.connect(self.close)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,首先导入了 `QLabel` 和 `QPushButton` 类。然后在 `MainWindow` 类的构造函数中,创建了一个标签和一个按钮,并使用 `move` 方法设置它们的位置。最后,将按钮的 `clicked` 信号连接到 `close` 槽上,以便在点击按钮时关闭窗口。
## 4. 布局管理器
在添加控件时,可以使用布局管理器来自动调整控件的位置和大小。常用的布局管理器包括垂直布局(`QVBoxLayout`)、水平布局(`QHBoxLayout`)和网格布局(`QGridLayout`)。
以下是一个使用水平布局和网格布局的 PyQt5 窗口程序:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QHBoxLayout, QGridLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 窗口程序")
self.setGeometry(100, 100, 400, 300)
# 创建容器
widget = QWidget()
self.setCentralWidget(widget)
# 创建布局管理器
hbox = QHBoxLayout()
grid = QGridLayout()
widget.setLayout(hbox)
# 添加标签和按钮到水平布局
label1 = QLabel('用户名:')
hbox.addWidget(label1)
edit1 = QLineEdit()
hbox.addWidget(edit1)
label2 = QLabel('密码:')
hbox.addWidget(label2)
edit2 = QLineEdit()
hbox.addWidget(edit2)
button1 = QPushButton('登录')
hbox.addWidget(button1)
button2 = QPushButton('退出')
hbox.addWidget(button2)
# 添加按钮到网格布局
button3 = QPushButton('1')
grid.addWidget(button3, 0, 0)
button4 = QPushButton('2')
grid.addWidget(button4, 0, 1)
button5 = QPushButton('3')
grid.addWidget(button5, 0, 2)
button6 = QPushButton('4')
grid.addWidget(button6, 1, 0)
button7 = QPushButton('5')
grid.addWidget(button7, 1, 1)
button8 = QPushButton('6')
grid.addWidget(button8, 1, 2)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,首先导入了 `QHBoxLayout`、`QGridLayout` 和 `QWidget` 类。然后创建了一个容器和两个布局管理器,并将容器设置为窗口的中心部件,并将水平布局添加到容器中。在水平布局中添加了标签、文本框和按钮。最后,将网格布局添加到容器中,并在网格布局中添加了一些按钮。
## 5. 事件处理
在 PyQt5 中,可以通过信号和槽来处理各种事件。信号是一种触发事件的机制,槽是一种响应事件的方法。可以使用 `connect` 方法将信号连接到槽上。
以下是一个使用信号和槽的 PyQt5 窗口程序:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QLineEdit, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt5 窗口程序")
self.setGeometry(100, 100, 400, 300)
# 添加标签和文本框
label1 = QLabel('用户名:', self)
label1.move(50, 50)
self.edit1 = QLineEdit(self)
self.edit1.move(150, 50)
label2 = QLabel('密码:', self)
label2.move(50, 100)
self.edit2 = QLineEdit(self)
self.edit2.move(150, 100)
# 添加按钮并连接信号和槽
button1 = QPushButton('登录', self)
button1.move(150, 150)
button1.clicked.connect(self.login)
button2 = QPushButton('退出', self)
button2.move(250, 150)
button2.clicked.connect(self.close)
# 登录槽函数
def login(self):
username = self.edit1.text()
password = self.edit2.text()
print('用户名:', username)
print('密码:', password)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,首先添加了两个标签和两个文本框。然后添加了两个按钮,并将第一个按钮的 `clicked` 信号连接到 `login` 槽上,将第二个按钮的 `clicked` 信号连接到 `close` 槽上。在 `login` 槽中,获取了文本框的内容,并打印出来。
相关推荐
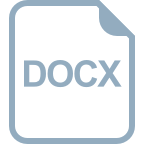














