spring boot security 跨域设置
时间: 2023-05-31 18:19:46 浏览: 182
### 回答1:
Spring Boot Security 跨域设置可以通过以下步骤实现:
1. 添加依赖
在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 配置跨域
在 Spring Boot Security 中,可以通过配置 CorsFilter 来实现跨域。在 WebSecurityConfigurerAdapter 中添加以下代码:
```
@Bean
public CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("*");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
return new CorsFilter(source);
}
```
以上代码中,我们允许所有来源、所有请求头、所有请求方法,并允许携带凭证。
3. 配置 Spring Security
在 WebSecurityConfigurerAdapter 中添加以下代码:
```
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and()
.httpBasic();
}
```
以上代码中,我们禁用了 CSRF 防护,并配置了 /api/** 路径需要认证才能访问。
4. 测试跨域
现在我们可以测试一下跨域是否生效。我们可以在前端页面中使用 Ajax 请求后端接口,例如:
```
$.ajax({
url: 'http://localhost:8080/api/test',
type: 'GET',
xhrFields: {
withCredentials: true
},
success: function(data) {
console.log(data);
},
error: function(xhr, status, error) {
console.log(error);
}
});
```
以上代码中,我们使用了 withCredentials 来允许携带凭证,这样就可以跨域访问后端接口了。
### 回答2:
跨域是web编程中常见的一个问题,它指的是一个网站请求访问另一个网站的资源时被浏览器所阻止。Spring Boot Security框架提供了一些已经包含在内的安全功能,包括认证、授权、防止跨站点请求伪造和防止跨站点脚本攻击等。
在Spring Boot Security跨域设置中,我们可以使用如下方式来实现:
1. 添加跨域设置的依赖项。
为了能够实现跨域请求,我们需要在Spring Boot应用程序的构建文件中添加跨域设置的依赖项。可以通过在pom.xml文件中添加如下代码来添加依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
```
这些依赖项可以让我们使用Spring Boot Security框架的功能。
2. 创建一个常规的Spring Boot配置类。
使用@Configuration注释创建Spring Boot配置类。这样做可以允许我们定义许多不同的Bean,在配置文件中可以将Bean打包在一起并供应用程序使用。我们可以使用以下代码创建一个简单的配置类。
```
@Configuration
public class CorsConfig {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedMethods("*");
}
};
}
}
```
3. 创建一个跨域请求处理器。
创建跨域请求处理对象,使用@CrossOrigin注释实现处理跨域。我们可以使用以下代码来实现这项功能。
```
@RestController
@RequestMapping("/api")
public class CorsController {
@CrossOrigin
@GetMapping("/test")
public String corsTest() {
return "CORS Testing";
}
}
```
通过以上方法,我们可以实现跨域访问资源,并且更好地保护我们的Web应用程序。
### 回答3:
Spring Boot是一个强大的Java框架,可以帮助构建RESTful API和Web应用程序。在Web应用程序的开发中,跨域请求是一个普遍的问题。这时候,Spring Boot Security可以帮助处理这个问题。
什么是跨域请求?
跨域请求指的是在一个域名下的网页获取另一个域名下的资源,而且该请求不能直接发出。
Spring Boot Security如何设置跨域请求?
在Spring Boot Security中通过配置CorsFilter来设置,CorsFilter会为跨域请求添加必要的头信息Access-Control-Allow-Origin,所以需要在SecurityConfig中进行CorsFilter的配置。
首先添加maven依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<version>2.4.5</version>
</dependency>
```
然后在SecurityConfig中新增CorsFilter:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter{
@Override
protected void configure(HttpSecurity http) throws Exception {
//关闭csrf跨站请求伪造
http.csrf().disable()
//处理跨域请求
.cors().and()
//配置权限
.authorizeRequests()
.antMatchers("/","/home","/index").permitAll()
//其他请求需要认证
.anyRequest().authenticated()
.and()
//允许注销登陆
.logout().permitAll();
}
@Bean
CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
//设置允许跨域的域名,*代表所有
configuration.setAllowedOrigins(Arrays.asList("*"));
//允许的请求方法
configuration.setAllowedMethods(Arrays.asList("OPTIONS", "GET", "POST", "PUT", "DELETE"));
//允许的头信息
configuration.setAllowedHeaders(Arrays.asList("Authorization", "Content-Type"));
//允许的cookie信息
configuration.setAllowCredentials(true);
//配置Url映射路径
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
```
这样就完成了Spring Boot Security的跨域设置,当然还需要跨域请求的测试。可以使用Postman或curl发起测试请求。如果测试请求正确返回结果,那么跨域请求已经实现了。
阅读全文
相关推荐
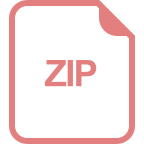
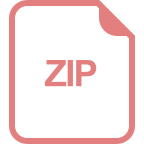
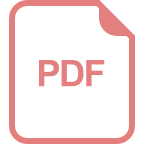
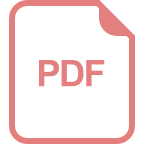
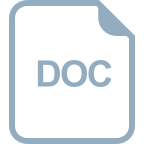
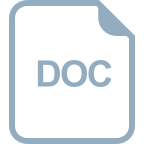
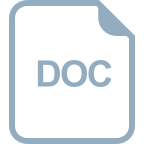
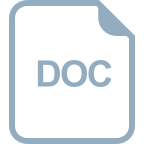
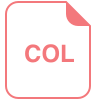
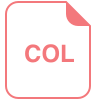
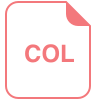
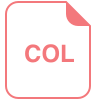
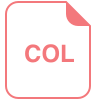
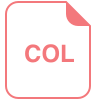

