TypeError: unsupported operand type(s) for -: 'tuple' and 'tuple'
时间: 2023-12-30 13:03:17 浏览: 128
这个错误通常发生在您尝试对两个元组进行减法操作时。例如,以下代码会导致该错误:
```
a = (1, 2, 3)
b = (4, 5, 6)
c = a - b
```
这是因为元组不支持减法操作。元组是不可变序列类型,意味着您不能更改元组中的元素或从中删除元素。因此,减法操作在元组中没有定义。
如果您想要执行元素级别的减法操作,可以使用 NumPy 库中的数组类型,例如:
```
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
c = a - b
```
这将产生一个包含每个元素的差异的新数组 c,即 [-3, -3, -3]。
相关问题
TypeError: unsupported operand type(s) for -: 'tuple' and 'int'
这个错误通常表示你正在尝试从一个元组中减去一个整数,这是不允许的。例如:
```
a = (1, 2, 3)
b = a - 1
```
以上代码就会报出 "TypeError: unsupported operand type(s) for -: 'tuple' and 'int'" 错误。
要解决这个问题,你需要检查你的代码并确定哪个元组中的值可能会被减去。你可以使用索引来访问元组中的特定元素,然后执行所需的操作。例如,如果你想从元组中的第一个元素中减去一个整数,可以像这样编写代码:
```
a = (1, 2, 3)
b = a[0] - 1
```
以上代码将从元组a的第一个元素中减去1,然后将结果存储在变量b中。
TypeError: unsupported operand type(s) for -: 'list' and 'tuple'
This error occurs when you try to subtract a tuple from a list or vice versa.
For example:
```
list1 = [1, 2, 3]
tuple1 = (4, 5, 6)
result = list1 - tuple1
```
This will raise a TypeError because you cannot subtract a tuple from a list.
To avoid this error, make sure you are performing operations between objects of the same type. If you need to convert one type to another, use the appropriate conversion function. For example, you can convert a list to a tuple using the `tuple()` function:
```
list1 = [1, 2, 3]
tuple1 = (4, 5, 6)
result = tuple(list1) - tuple1
```
阅读全文
相关推荐
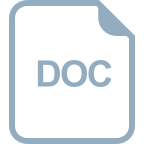
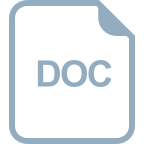
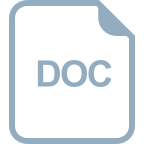










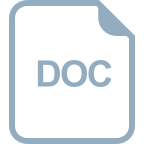
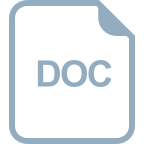
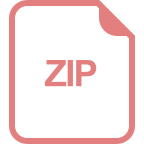
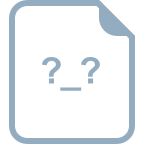