定义一个模板类Student,为了增强类的适用性,将学号设计为参数化类型,它可以实例化成字符串、长整形等;将成绩设计为参数化类型,它可以实例化成整形、浮点型、字符型(用来表示等级分)等。 template<class TNo, class TScore, int num>//TNo和TScore为参数化类型 class Student{ private: TNo StudentID; //参数化类型,存储姓名 TScore score[num]; //参数化类型数组,存储num门课程的分数 public: void Input();//数据的录入 TScore MaxScore(); //查找score的最大值并返回该值 void Update(TScore sscore,int i);//更新学生的第i门课程成绩为sscore void SelectSort(); //采用选择排序法对学生成绩进行升序排列 void Print(); //输出所有学生的信息 }; 请注意String是自定义的class类型,参考如下: class String{ public: char Str[20]; friend istream &operator>>(istream &in, String &s); friend ostream &operator<<(ostream &out, String &s); }; 请自行设计主函数,并做如下声明。从键盘依次录入数据,然后分别调用四个成员函数执行得到所需的结果。 Student<String,float,3>;
时间: 2024-02-28 08:54:02 浏览: 118
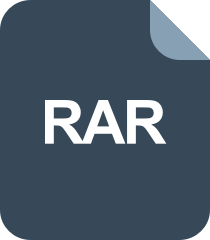
建立一个名为Student的类,该类有以下几个私有成员变量:学生姓名、学号、性别、年龄。还有以下两个成员函数:一个用于初始化学生姓名、学号、性别和年龄的构造函数,一个用于输出学生信息的函数。编写一个主函数。声明一个学学生对象。然后调用成员函数在屏幕输出学生信息。

以下是实现了上述要求的代码:
```
#include<iostream>
using namespace std;
class String{
public:
char Str[20];
friend istream &operator>>(istream &in, String &s);
friend ostream &operator<<(ostream &out, String &s);
};
istream &operator>>(istream &in, String &s){
in>>s.Str;
return in;
}
ostream &operator<<(ostream &out, String &s){
out<<s.Str;
return out;
}
template<class TNo, class TScore, int num>
class Student{
private:
TNo StudentID;
TScore score[num];
public:
void Input(){
cout<<"请输入学生姓名:";
cin>>StudentID;
cout<<"请输入学生"<<num<<"门课程的成绩:";
for(int i=0;i<num;i++){
cin>>score[i];
}
}
TScore MaxScore(){
TScore maxScore=score[0];
for(int i=1;i<num;i++){
if(score[i]>maxScore){
maxScore=score[i];
}
}
return maxScore;
}
void Update(TScore sscore,int i){
score[i-1]=sscore;
cout<<"更新后的学生信息为:"<<endl;
Print();
}
void SelectSort(){
for(int i=0;i<num-1;i++){
int minIndex=i;
for(int j=i+1;j<num;j++){
if(score[j]<score[minIndex]){
minIndex=j;
}
}
if(minIndex!=i){
TScore temp=score[i];
score[i]=score[minIndex];
score[minIndex]=temp;
}
}
cout<<"排好序的学生信息为:"<<endl;
Print();
}
void Print(){
cout<<StudentID<<"\t";
for(int i=0;i<num;i++){
cout<<score[i]<<"\t";
}
cout<<endl;
}
};
int main(){
Student<String,float,3> stu;
stu.Input();
cout<<"该学生最高分为:"<<stu.MaxScore()<<endl;
stu.Update(90,2);
stu.SelectSort();
return 0;
}
```
在主函数中,我们实例化了一个参数为String、TScore为float、num为3的Student类对象stu,并依次调用了Input、MaxScore、Update、SelectSort和Print函数进行数据录入、求最高分、更新成绩、排序和输出所有学生信息的操作。
阅读全文
相关推荐
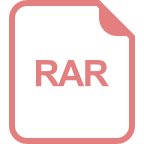
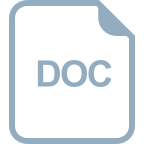
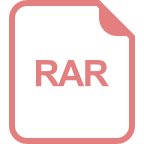
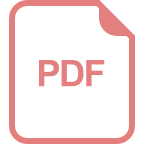
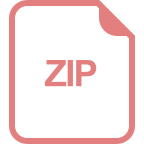
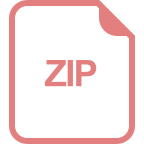