用httpclient 写一个post请求,请求发送json返回json
时间: 2024-01-30 17:43:19 浏览: 85
以下是使用Apache HttpComponents的HttpClient库发送POST请求并获取响应的示例代码:
```java
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
public class HttpClientExample {
public static void main(String[] args) {
String url = "http://example.com/api";
// 构造POST请求
HttpPost post = new HttpPost(url);
post.setHeader("Content-Type", "application/json");
// 构造JSON请求体
String requestBody = "{\"username\":\"johndoe\",\"password\":\"secret\"}";
StringEntity entity = new StringEntity(requestBody, ContentType.APPLICATION_JSON);
post.setEntity(entity);
// 发送POST请求
try (CloseableHttpClient httpClient = HttpClientBuilder.create().build();
CloseableHttpResponse response = httpClient.execute(post)) {
// 获取响应实体
HttpEntity responseEntity = response.getEntity();
String responseBody = responseEntity != null ? EntityUtils.toString(responseEntity, StandardCharsets.UTF_8) : "";
// 输出响应信息
System.out.println("HTTP Status Code: " + response.getStatusLine().getStatusCode());
System.out.println("Response Body:\n" + responseBody);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在以上示例代码中,我们使用了HttpPost类来构造POST请求,设置了请求头Content-Type为application/json,并且构造了一个JSON请求体。然后使用HttpClient发送POST请求,并获取响应实体,最后输出响应信息。
需要注意的是,以上示例代码中使用了try-with-resources语句,确保HttpClient和HttpResponse对象在使用完毕后自动关闭。
阅读全文
相关推荐
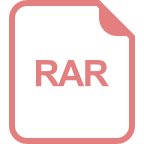
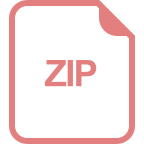
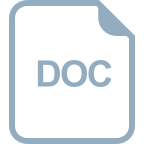
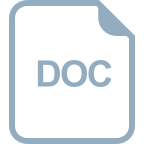










