如何判断三个值在 IEnumerable<String>里面都存在
时间: 2024-02-05 11:10:33 浏览: 24
可以使用 LINQ 的 `All` 方法,它接受一个 lambda 表达式作为参数,用于判断集合中的所有元素是否都满足某个条件。
示例代码:
```csharp
IEnumerable<string> strings = new[] { "foo", "bar", "baz" };
string[] valuesToCheck = { "foo", "bar", "qux" };
bool allExist = valuesToCheck.All(value => strings.Contains(value));
```
在这个示例中,`valuesToCheck` 数组中的所有值都会被检查,如果它们都存在于 `strings` 集合中,则 `allExist` 变量的值为 `true`,否则为 `false`。
相关问题
wpf 如何判断对象集合里面包含某个属性值
要判断一个对象集合是否包含某个属性值,可以使用 LINQ 查询语句和反射来实现。具体实现步骤如下:
1. 使用 LINQ 查询语句从对象集合中筛选出包含指定属性的对象。
2. 使用反射获取对象的属性列表。
3. 遍历对象集合中的每个对象,判断对象的指定属性值是否等于目标值。
4. 如果对象包含指定属性值,则返回 true,否则返回 false。
以下是示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
class Program
{
static void Main(string[] args)
{
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 28 },
new Person { Name = "Bob", Age = 30 },
new Person { Name = "Charlie", Age = 35 },
};
bool hasAge30 = HasPropertyValue(people, "Age", 30);
Console.WriteLine("Has Age=30 property value: " + hasAge30);
bool hasAge40 = HasPropertyValue(people, "Age", 40);
Console.WriteLine("Has Age=40 property value: " + hasAge40);
Console.ReadKey();
}
static bool HasPropertyValue(IEnumerable<object> collection, string propertyName, object propertyValue)
{
var type = collection.GetType().GetGenericArguments()[0];
var properties = type.GetProperties();
var property = properties.FirstOrDefault(p => p.Name == propertyName);
if (property == null)
{
return false;
}
return collection.Any(obj => property.GetValue(obj) == propertyValue);
}
}
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
```
在上面的示例中,我们定义了一个包含 Name 和 Age 两个属性的 Person 类,并创建了一个包含三个 Person 对象的 List。然后,我们分别判断该 List 是否包含 Age=30 和 Age=40 两个属性值。最终输出结果为:
```
Has Age=30 property value: True
Has Age=40 property value: False
```
可以看到,HasPropertyValue 方法可以判断对象集合是否包含指定的属性值。
c# 如何判断对象集合里面包含某个属性值
要判断一个对象集合是否包含某个属性值,可以使用 LINQ 查询语句和反射来实现。具体实现步骤如下:
1. 使用 LINQ 查询语句从对象集合中筛选出包含指定属性的对象。
2. 使用反射获取对象的属性列表。
3. 遍历对象集合中的每个对象,判断对象的指定属性值是否等于目标值。
4. 如果对象包含指定属性值,则返回 true,否则返回 false。
以下是示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
class Program
{
static void Main(string[] args)
{
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 28 },
new Person { Name = "Bob", Age = 30 },
new Person { Name = "Charlie", Age = 35 },
};
bool hasAge30 = HasPropertyValue(people, "Age", 30);
Console.WriteLine("Has Age=30 property value: " + hasAge30);
bool hasAge40 = HasPropertyValue(people, "Age", 40);
Console.WriteLine("Has Age=40 property value: " + hasAge40);
Console.ReadKey();
}
static bool HasPropertyValue(IEnumerable<object> collection, string propertyName, object propertyValue)
{
var type = collection.GetType().GetGenericArguments()[0];
var properties = type.GetProperties();
var property = properties.FirstOrDefault(p => p.Name == propertyName);
if (property == null)
{
return false;
}
return collection.Any(obj => property.GetValue(obj) == propertyValue);
}
}
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
```
在上面的示例中,我们定义了一个包含 Name 和 Age 两个属性的 Person 类,并创建了一个包含三个 Person 对象的 List。然后,我们分别判断该 List 是否包含 Age=30 和 Age=40 两个属性值。最终输出结果为:
```
Has Age=30 property value: True
Has Age=40 property value: False
```
可以看到,HasPropertyValue 方法可以判断对象集合是否包含指定的属性值。
相关推荐
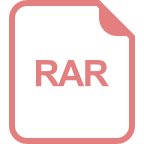
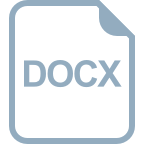
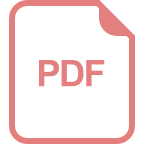












