图书管理系统借书还书c++
时间: 2023-12-02 17:02:05 浏览: 50
以下是C++图书管理系统中借书还书的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义图书结构体
struct Book {
string name; // 书名
string author; // 作者
string publisher; // 出版社
string ISBN; // ISBN号
bool borrowed; // 是否被借出
string borrower; // 借阅人
};
// 借书函数
void borrowBook(Book &book, string borrower) {
if (book.borrowed) {
cout << "该书已被借出!" << endl;
} else {
book.borrowed = true;
book.borrower = borrower;
cout << "借书成功!" << endl;
}
}
// 还书函数
void returnBook(Book &book) {
if (book.borrowed) {
book.borrowed = false;
book.borrower = "";
cout << "还书成功!" << endl;
} else {
cout << "该书未被借出!" << endl;
}
}
int main() {
// 读取图书信息
ifstream fin("books.txt");
int bookCount;
fin >> bookCount;
Book *books = new Book[bookCount];
for (int i = 0; i < bookCount; i++) {
fin >> books[i].name >> books[i].author >> books[i].publisher >> books[i].ISBN >> books[i].borrowed >> books[i].borrower;
}
fin.close();
// 借书还书
string name, borrower;
cout << "请输入要借阅的书名:";
cin >> name;
bool found = false;
for (int i = 0; i < bookCount; i++) {
if (books[i].name == name) {
found = true;
if (books[i].borrowed) {
cout << "该书已被借出,借阅人为:" << books[i].borrower << endl;
cout << "请输入还书人姓名:";
cin >> borrower;
if (books[i].borrower == borrower) {
returnBook(books[i]);
} else {
cout << "还书人姓名错误! << endl;
}
} else {
cout << "请输入借书人姓名:";
cin >> borrower;
borrowBook(books[i], borrower);
}
break;
}
}
if (!found) {
cout << "未找到该书!" << endl;
}
// 保存图书信息
ofstream fout("books.txt");
fout << bookCount << endl;
for (int i = 0; i < bookCount; i++) {
fout << books[i].name << " " << books[i].author << " " << books[i].publisher << " " << books[i].ISBN << " " << books[i].borrowed << " " << books[i].borrower << endl;
}
fout.close();
delete[] books;
return 0;
}
```
相关推荐
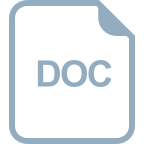
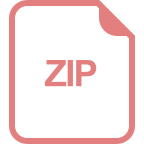
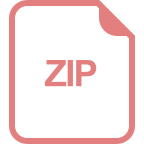














