在react类组件使用handsonTable/react组件时怎么根据hotInstance.table.clientWidth来更新表格的width
时间: 2024-09-11 12:14:24 浏览: 67
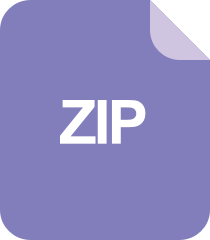
基于react.js的组件化3d模型查看工具
在React类组件中使用handsontable/react组件时,如果你想根据`hotInstance.table.clientWidth`的值来动态更新表格的宽度,你可以通过以下步骤实现:
1. 首先,确保你在组件中正确地创建并引用了handsontable实例。通常,这可以通过在组件的`componentDidMount`生命周期方法中初始化handsontable,并将其赋值给一个实例变量来完成。
2. 接下来,你需要添加一个事件监听器,监听窗口的`resize`事件或者其他可能影响表格宽度的事件。这样可以在相关尺寸变化时进行响应。
3. 在事件监听器的回调函数中,你可以获取当前表格的实例,然后读取`table.clientWidth`属性来获取当前表格的宽度。
4. 最后,将获取到的宽度值应用到表格组件上。这通常意味着你需要在表格的状态中更新一个表示宽度的字段,并在表格组件的`render`方法中使用这个字段来设置表格的宽度样式。
下面是一个简化的代码示例:
```jsx
import React, { Component } from 'react';
import { HotTable } from 'handsontable/react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.hotInstance = null;
this.state = {
width: 'auto' // 初始宽度
};
}
componentDidMount() {
const hotContainer = document.getElementById('hot-table-container');
this.hotInstance = new Handsontable(hotContainer, {
// ...其他配置项
});
this.updateTableWidth();
window.addEventListener('resize', this.updateTableWidth);
}
componentWillUnmount() {
window.removeEventListener('resize', this.updateTableWidth);
}
updateTableWidth = () => {
const newWidth = this.hotInstance.table.clientWidth;
this.setState({ width: `${newWidth}px` });
}
render() {
return (
<HotTable
ref={el => this.hotTable = el}
data={...}
width={this.state.width}
// ...其他props
/>
);
}
}
// 渲染到指定的容器中
const hotTableContainer = document.getElementById('hot-table-container');
ReactDOM.render(<MyComponent />, hotTableContainer);
```
在上面的代码中,`updateTableWidth`方法会在组件挂载后立即调用,并且在窗口大小改变时被调用,来更新表格的宽度。
阅读全文
相关推荐
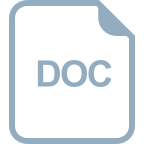
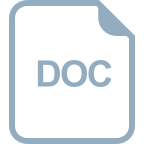






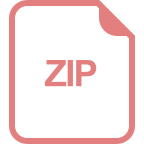
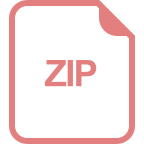
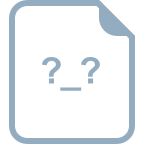
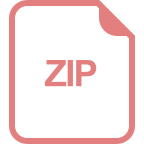
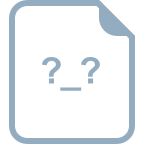
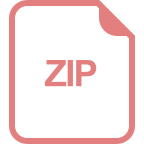
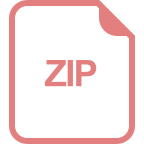
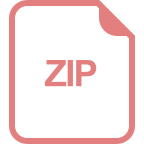
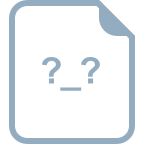
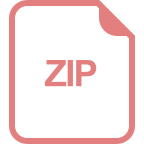