设计一个c语言算法并给出测试程序,统计在输入字符中各个不同字符出现的频度并将结果存入文件(字符串中的合法字符为A~Z这26个字母和0~9这10个数字)。
时间: 2024-10-21 19:15:39 浏览: 6
设计一个C语言算法来统计输入字符中每个不同字符及其频率,并将结果存储到文件中,可以分为以下几个步骤:
1. **创建主函数**:接收用户输入的字符串,初始化字符计数数组,打开文件用于写入。
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_CHAR 36 // 总共考虑26个英文字母 + 10个数字
// 结构体表示字符和其出现次数
typedef struct {
char ch;
int count;
} CharCount;
void count_chars(char *input, FILE *file);
```
2. **count_chars 函数**:遍历输入字符串,更新字符计数并写入文件。
```c
void count_chars(char *input, FILE *file) {
CharCount counts[MAX_CHAR];
memset(counts, 0, sizeof(counts));
for (int i = 0; input[i] != '\0'; ++i) {
if (isalnum(input[i])) { // 检查是否是字母或数字
counts[input[i]-'A' + '0']++; // 根据ASCII值计算索引并增加计数
}
}
for (int j = 0; j < MAX_CHAR; ++j) {
if (counts[j]) {
fprintf(file, "%c occurs %d times.\n", 'A'+j, counts[j]);
}
}
}
```
3. **主程序**:获取用户输入、创建文件、调用函数并关闭文件。
```c
int main() {
char input[100];
FILE *output_file = fopen("char_frequency.txt", "w");
if (!output_file) {
printf("Error opening file.\n");
return 1;
}
printf("Enter a string of alphanumeric characters: ");
fgets(input, sizeof(input), stdin); // Don't include newline
input[strcspn(input, "\n")] = '\0'; // Remove newline if present
count_chars(input, output_file);
fclose(output_file);
return 0;
}
```
**测试程序**:运行上述`main`函数,输入一个包含A-Z和0-9的字符串,程序会生成一个名为"char_frequency.txt"的文件,其中列出了每种字符及其出现次数。
阅读全文
相关推荐
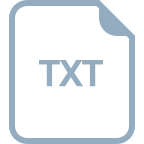
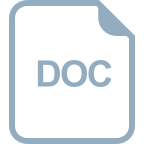
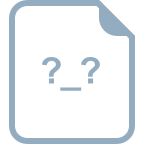
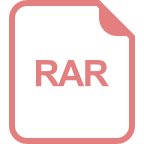
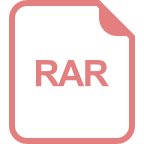
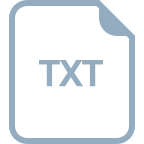
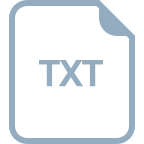
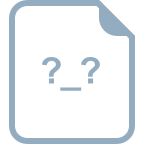
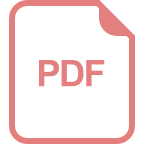
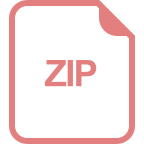
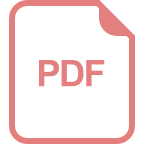
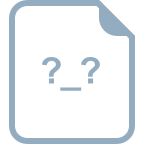
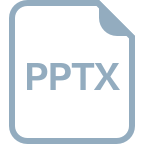