springboot 邮件框架
时间: 2023-08-30 20:08:13 浏览: 60
Spring Boot提供了一个简单而强大的邮件框架,可以轻松地在应用程序中发送电子邮件。下面是使用Spring Boot发送电子邮件的基本步骤:
1. 添加依赖:在你的项目的pom.xml文件中添加Spring Boot的邮件依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
2. 配置邮件属性:在application.properties(或application.yml)文件中配置SMTP(Simple Mail Transfer Protocol)服务器的相关属性。示例配置如下:
```properties
spring.mail.host=your-smtp-server
spring.mail.port=your-smtp-port
spring.mail.username=your-username
spring.mail.password=your-password
```
3. 创建邮件服务类:创建一个Java类,用于发送电子邮件。可以使用JavaMailSender类来发送电子邮件。示例如下:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender javaMailSender;
public void sendEmail(String to, String subject, String body) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(body);
javaMailSender.send(message);
}
}
```
4. 在需要发送邮件的地方使用邮件服务类:在你的代码中,使用@Autowired注解将邮件服务类注入到需要发送邮件的地方,并调用sendEmail方法来发送邮件。示例代码如下:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class EmailController {
@Autowired
private EmailService emailService;
@GetMapping("/sendEmail")
public String sendEmail() {
String to = "recipient@example.com";
String subject = "Test Email";
String body = "This is a test email.";
emailService.sendEmail(to, subject, body);
return "Email sent successfully.";
}
}
```
这是一个简单的使用Spring Boot发送电子邮件的示例。你可以根据自己的需求进行进一步的定制和扩展。
相关推荐
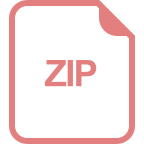














