输入一行字符串分别计算出其中英文字母空格数字和其他字符的个数。
时间: 2024-11-09 08:31:20 浏览: 18
为了计算输入字符串中英文字母、空格、数字以及其他字符的数量,你可以编写一个简单的程序,通常使用编程语言的内置函数来遍历字符串并统计各个类别。以下是一个Python示例:
```python
def count_characters(s):
alpha_count = sum(1 for c in s if c.isalpha()) # 英文字母
space_count = s.count(' ') # 空格
digit_count = sum(1 for c in s if c.isdigit()) # 数字
other_count = len(s) - (alpha_count + space_count + digit_count) # 其他字符(通过总长度减去已知字符的数量)
return alpha_count, space_count, digit_count, other_count
# 示例
input_string = "Hello, World! This is a test string with 123 numbers."
alpha, space, digit, other = count_characters(input_string)
print(f"字母: {alpha}, 空格: {space}, 数字: {digit}, 其他字符: {other}")
#
相关问题
输入一行字符,分别统计出其中英文字母空格数字和其他字符的个数。
在程序设计中,统计输入字符串中各组成部分的数量可以使用循环遍历和条件判断来实现。下面是一个简单的Python示例:
```python
def count_chars(input_str):
alpha_count = 0
space_count = 0
digit_count = 0
other_count = 0
for char in input_str:
if char.isalpha(): # 检查是否为字母
alpha_count += 1
elif char.isspace(): # 检查是否为空格
space_count += 1
elif char.isdigit(): # 检查是否为数字
digit_count += 1
else: # 其他字符(非字母、空格和数字)
other_count += 1
return alpha_count, space_count, digit_count, other_count
input_string = input("请输入一行字符: ")
result = count_chars(input_string)
print(f"英文字母数量: {result[0]}")
print(f"空格数量: {result[1]}")
print(f"数字数量: {result[2]}")
print(f"其他字符数量: {result[3]}")
python中输入一行字符串,分别统计其中英文字母 空格 数字和其它字符的个数
好的,下面是 Python 代码实现这个功能:
```python
s = input("请输入一行字符串:") # 获取用户输入的字符串
count_letter = 0 # 统计英文字母的个数
count_space = 0 # 统计空格的个数
count_digit = 0 # 统计数字的个数
count_other = 0 # 统计其他字符的个数
for c in s: # 遍历字符串中的每个字符
if c.isalpha(): # 如果是英文字母
count_letter += 1
elif c.isspace(): # 如果是空格
count_space += 1
elif c.isdigit(): # 如果是数字
count_digit += 1
else: # 否则是其他字符
count_other += 1
print("英文字母个数:", count_letter)
print("空格个数:", count_space)
print("数字个数:", count_digit)
print("其他字符个数:", count_other)
```
你可以直接复制这段代码到 Python 环境中运行。输入一行字符串,程序会统计其中英文字母、空格、数字和其他字符的个数,并输出结果。
阅读全文
相关推荐
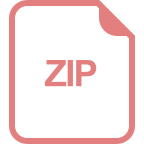
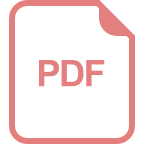













