Vue3 defineModel 传递数组
时间: 2024-07-22 17:00:52 浏览: 111
Vue3中的`defineModel`是响应式模型(Reactive Model)的一部分,用于创建和管理复杂的绑定状态。当你需要处理数组数据时,可以使用它来监听数组的变化并进行相应操作。
例如,你可以这样做:
```javascript
import { ref, onBeforeUpdate } from 'vue';
const items = ref([]);
onBeforeUpdate(() => {
// 检查items是否进行了增删改操作
if (Array.isArray(items.value) && !_.isEqual(items.oldValue, items.value)) {
// 在这里处理数组变化,如更新列表、触发其他计算等
console.log('Items updated:', items.value);
}
});
// 使用defineModel的数组版本,如设置或获取数组元素
const addItem = (item) => {
items.value.push(item);
};
const removeItem = (index) => {
items.value.splice(index, 1);
};
```
`items.value`是一个响应式的数组,每次修改都会自动触发依赖更新。你可以使用`push`, `pop`, `splice`等方法操作这个数组,并通过`onBeforeUpdate`钩子来监听变动。
相关问题
vue向后端传递数组
在Vue中,可以通过axios库向后端传递数组。
例如,如果要向后端传递一个名为“ids”的数组,可以按照以下步骤操作:
1. 在Vue组件中定义ids数组,并初始化为空数组。
```javascript
data() {
return {
ids: []
}
},
```
2. 在模板中定义一个表单,包含一个文本框和一个提交按钮,用来输入和提交ids数组。
```html
<template>
<form @submit.prevent="submitIds">
<input type="text" v-model="idStr">
<button type="submit">提交</button>
</form>
</template>
```
3. 在Vue组件中定义一个提交函数submitIds,该函数使用axios库向后端传递ids数组。
```javascript
methods: {
submitIds() {
// 将idStr字符串转换为数组,以逗号为分隔符
this.ids = this.idStr.split(',');
// 向后端传递ids数组
axios.post('/api/ids', {
ids: this.ids
}).then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
}
},
```
其中,axios.post()方法用于向后端发送POST请求,第一个参数是请求的URL,第二个参数是请求的数据,该数据包含一个名为“ids”的数组,其值为Vue组件中定义的ids数组。
注意,在上述代码中,我们将从文本框中获取的idStr字符串转换为数组,以逗号为分隔符。这是因为在提交表单时,文本框中输入的是字符串,需要将其转换为数组才能传递给后端。
vue3 父子组件传递数组
在 Vue3 中,可以通过 props 将数组从父组件传递到子组件。具体步骤如下:
1. 在父组件中定义一个数组,并将其作为 props 传递给子组件:
```html
<template>
<div>
<child-component :myArray="parentArray"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
parentArray: [1, 2, 3],
};
},
};
</script>
```
2. 在子组件中,通过 props 接收父组件传递的数组,并在模板中使用:
```html
<template>
<div>
<ul>
<li v-for="item in myArray" :key="item">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
props: {
myArray: {
type: Array,
required: true,
},
},
};
</script>
```
这样就可以在子组件中使用父组件传递的数组了。
相关推荐
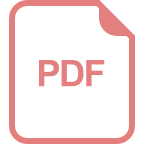
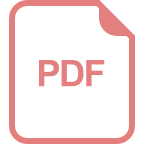
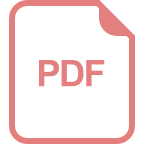












