sizeof怎么对字符串运算
时间: 2024-11-25 12:09:29 浏览: 6
`sizeof` 是 C 语言中的一个运算符,用于计算某个数据类型或变量所占用的内存字节数。当你想要获取字符串(`char`数组)的大小时,你应该用 `sizeof` 运算符直接放在字符串常量、字符数组或指针上。
例如:
```c
char str[] = "Hello, World!";
size_t len = sizeof(str); // 获取整个数组的大小,包括终止符'\0'
// 或者如果你有一个指向字符串的指针
const char* ptr = "Hello";
size_t len = strlen(ptr); // 使用 strlen 函数得到实际字符的数量,但不要忘记加上 sizeof(char),因为每个字符占一个字节
// 注意,strlen 只返回可打印字符(不包括终止符)的数量,如果你想包括终止符,用 sizeof 还是比较直观的。
```
需要注意的是,`sizeof` 对于空字符串(只包含终止符 '\0')的长度也会返回 1,因为它也需要一个字节来存储终止符。如果字符串不是以 '\0' 结尾,或者是指针而不是数组,你需要额外处理这种情况。
相关问题
链式串求字符串的长度的运算c语言
链式存储的字符串在C语言中通常使用字符指针数组或动态分配的结构体(如`char*`或`struct Node`)来表示,每个节点包含一个字符和指向下一个节点的指针。若直接通过链表计算长度,你需要遍历整个链表,直到遇到空字符'\0'为止。
下面是一个简单的示例,展示了如何通过链式结构计算字符串长度:
```c
#include <stdio.h>
#include <string.h>
// 定义链表节点结构
typedef struct Node {
char data;
struct Node* next;
} Node;
// 初始化链表节点
Node* createNode(char ch) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = ch;
newNode->next = NULL;
}
return newNode;
}
// 计算链式字符串长度
int strLength(Node* head) {
int length = 0;
while (head != NULL) {
length++;
head = head->next;
}
return length;
}
int main() {
// 创建链式字符串,例如 "Hello, World!"
Node* head = createNode('H');
head->next = createNode('e');
head->next->next = createNode('l');
// ... 继续添加其他字符 ...
int len = strLength(head);
printf("The length of the string is: %d\n", len);
return 0;
}
```
在这个例子中,`strLength`函数通过遍历链表,逐个增加计数器`length`的值,直到遍历到链表结束。
用C语言怎么判断不同字符串执行不同的运算
在C语言中,你可以通过函数指针或结构体结合动态内存分配来实现根据不同字符串执行不同的运算。这里有一个简单的例子:
1. 函数指针:定义一系列指向运算函数的指针,每个函数对应一种运算。例如,你可以有`add`, `subtract`, `multiply`, `divide`等函数。
```c
#include <stdio.h>
#include <stdlib.h>
void add(const char* str) {
// 实现加法运算
}
void subtract(const char* str) {
// 实现减法运算
}
// ... 其他运算函数
int main() {
void (*op)(const char*) = NULL; // 定义函数指针
scanf("Enter operation: %s", &str); // 用户输入运算符
switch (str[0]) {
case '+':
op = add;
break;
case '-':
op = subtract;
break;
// 添加更多case处理其他运算符
default:
printf("Invalid operator.\n");
return 1;
}
if (op != NULL) {
// 调用对应的函数
op(str);
} else {
printf("No operation provided.\n");
}
return 0;
}
```
2. 结构体和函数指针数组:如果字符串代表的是某种操作的名称,可以将操作作为结构体的一部分,然后存储在数组中。
```c
typedef struct {
const char* name;
void (*func)(const char*);
} Operation;
Operation operations[] = {
{"+", add},
{"-", subtract},
// ... 其他运算结构
};
main() {
char input[256];
scanf("%s", input);
for (size_t i = 0; i < sizeof(operations) / sizeof(Operation); ++i) {
if (!strcmp(input, operations[i].name)) {
operations[i].func(input);
break;
}
}
// ...后续处理
}
```
阅读全文
相关推荐
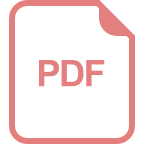
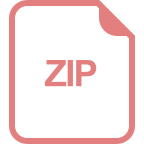
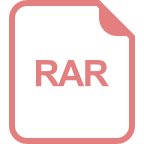
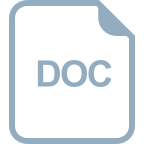
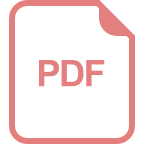
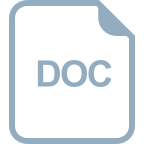
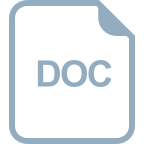









