输入十个字符串,要求从小到大排序输出。用c语言
时间: 2024-12-21 09:23:18 浏览: 5
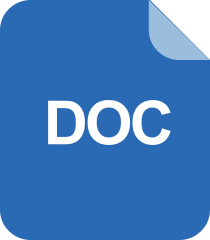
按照字符串顺序从小到大排序,删除重复字符
在C语言中,我们可以使用标准库函数`qsort()`来进行数组元素的排序,包括字符串。以下是一个简单的示例,假设你有名为`strs[]`的数组,它存储了你要排序的十个字符串:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义字符串比较函数,用于qsort
int compare(const void *a, const void *b) {
return strcmp(*(const char **)a, *(const char **)b);
}
void sort_strings(char *strs[], int n) {
qsort(strs, n, sizeof(char*), compare);
}
int main() {
char strs[10][50] = {"hello", "world", "apple", "banana", "zebra", "cat", "dog", "elephant", "fox", "giraffe"};
int num_strings = sizeof(strs) / sizeof(strs[0]);
// 排序字符串
sort_strings(strs, num_strings);
// 输出排序后的字符串
for (int i = 0; i < num_strings; ++i) {
printf("%s ", strs[i]);
}
printf("\n");
return 0;
}
```
这个程序首先定义了一个比较函数`compare`,然后在`main`函数中创建一个字符串数组并调用`sort_strings`对其进行排序。最后,通过循环打印出排序后的字符串。
注意,由于C语言字符串是以'\0'结尾的,所以这里假设每个字符串都在一个单独的数组元素中。
阅读全文
相关推荐















