linux i2c读写atc24 eeprom代码
时间: 2024-02-09 08:10:44 浏览: 156
以下是一个简单的在Linux系统下使用I2C协议读写AT24CXX系列EEPROM的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <linux/i2c-dev.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#define I2C_ADDR 0x50 // EEPROM的I2C地址
#define EEPROM_SIZE 256 // EEPROM的大小
int main(void)
{
int fd;
char buf[EEPROM_SIZE], data[EEPROM_SIZE];
int i;
// 打开I2C总线
if ((fd = open("/dev/i2c-1", O_RDWR)) < 0) {
perror("Failed to open i2c bus");
exit(1);
}
// 设置从设备地址
if (ioctl(fd, I2C_SLAVE, I2C_ADDR) < 0) {
perror("Failed to set i2c address");
exit(1);
}
// 读EEPROM
if (read(fd, buf, EEPROM_SIZE) != EEPROM_SIZE) {
perror("Failed to read from the i2c bus");
exit(1);
}
// 打印EEPROM中的数据
printf("Read data: ");
for (i = 0; i < EEPROM_SIZE; i++) {
printf("%02x ", buf[i]);
}
printf("\n");
// 写EEPROM
for (i = 0; i < EEPROM_SIZE; i++) {
data[i] = i & 0xff;
}
if (write(fd, data, EEPROM_SIZE) != EEPROM_SIZE) {
perror("Failed to write to the i2c bus");
exit(1);
}
// 读EEPROM
if (read(fd, buf, EEPROM_SIZE) != EEPROM_SIZE) {
perror("Failed to read from the i2c bus");
exit(1);
}
// 打印EEPROM中的数据
printf("Read data: ");
for (i = 0; i < EEPROM_SIZE; i++) {
printf("%02x ", buf[i]);
}
printf("\n");
// 关闭I2C总线
close(fd);
return 0;
}
```
在使用该代码之前,需要确保已经将I2C总线驱动加载到内核中,并且已经连接好了AT24CXX系列EEPROM。如果需要使用其他I2C从设备,只需要将I2C_ADDR修改为对应从设备的地址即可。
阅读全文
相关推荐


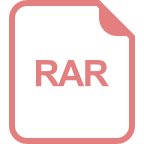














