python实现自由落体功能效果实现
时间: 2023-10-29 21:04:42 浏览: 81
可以使用以下代码实现自由落体的效果:
```python
import time
g = 9.8 # 重力加速度,单位为 m/s^2
h = float(input("请输入初始高度(单位为米):"))
t = 0 # 时间,单位为秒
v = 0 # 初始速度为0,单位为 m/s
while h >= 0:
print("时间:{:.2f}秒,高度:{:.2f}米".format(t, h))
t += 0.1
v += g * 0.1
h -= v * 0.1
time.sleep(0.1)
```
运行以上代码,在控制台中输入初始高度(单位为米),即可开始模拟自由落体的过程。程序会每隔0.1秒输出当前时间和高度,并且不断更新高度和速度的值,直到物体落地为止。
相关问题
python球自由落体
好的,关于 Python 实现自由落体的问题,可以通过以下代码实现:
```python
import math
def free_fall(time, initial_velocity=0, acceleration=9.8):
final_velocity = initial_velocity + acceleration * time
distance = initial_velocity * time + 0.5 * acceleration * math.pow(time, 2)
return final_velocity, distance
```
其中,`time` 表示自由落体的时间,`initial_velocity` 表示初始速度(默认为 0),`acceleration` 表示加速度(默认为 9.8)。
例如,如果要计算自由落体 3 秒钟后的速度和位移,可以这样调用函数:
```python
final_velocity, distance = free_fall(3)
print("Final velocity:", final_velocity)
print("Distance:", distance)
```
输出结果为:
```
Final velocity: 29.4
Distance: 44.1
```
python代码自由落体运动
自由落体是指在没有空气阻力的情况下,物体在重力作用下自由下落的运动。下面是Python实现自由落体运动的代码:
```python
h = float(input("请输入初始高度(米):"))
t = float(input("请输入时间间隔(秒):"))
g = 9.8 # 重力加速度
# 计算自由落体运动的高度
while h > 0:
print("当前高度为:{:.2f}米".format(h))
h = h - 0.5 * g * t ** 2
```
代码中,首先通过`input`函数获取初始高度和时间间隔,然后利用`while`循环计算自由落体运动的高度,直到物体落地为止。在循环中,每次计算当前高度并输出,然后更新高度值。需要注意的是,这里假设没有空气阻力,因此重力加速度取常数9.8米/秒^2。
相关推荐
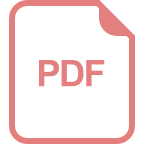












