实现发送邮件的Java详细代码
时间: 2024-05-08 08:18:17 浏览: 9
以下是一个简单的实现发送邮件的Java代码示例:
```java
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class EmailSender {
public static void main(String[] args) {
final String username = "your_email_address@gmail.com"; // 发送邮件的邮箱账号
final String password = "your_email_password"; // 发送邮件的邮箱密码
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com"); // 发件人邮箱的 SMTP 服务器地址
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your_email_address@gmail.com")); // 发送人邮箱地址
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse("recipient_email_address@gmail.com")); // 接收邮件的邮箱地址
message.setSubject("Testing Subject"); // 邮件主题
message.setText("Dear Mail Crawler,"
+ "\n\n No spam to my email, please!"); // 邮件内容
Transport.send(message);
System.out.println("Email sent successfully!");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
```
请注意,您需要将这些代码中的“your_email_address”和“your_email_password”替换为您自己的邮箱地址和密码,并根据您的实际情况修改其他必要的参数。此外,您还需要在发送邮件之前在您的邮箱账户中启用SMTP功能。
相关推荐
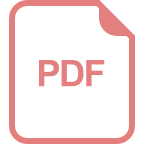














