Python模拟康普顿散射
时间: 2023-12-14 19:33:20 浏览: 43
康普顿散射是指入射光子与物质中的自由电子发生非弹性碰撞后,光子的能量和方向发生改变的过程。下面是一个简单的Python模拟康普顿散射的例子:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义常量
m_e = 9.10938356e-31 # 电子质量
c = 299792458 # 光速
h = 6.62607015e-34 # 普朗克常数
lambda_0 = 1e-10 # 入射光波长
E_0 = h * c / lambda_0 # 入射光子能量
# 定义函数
def compton_scatter(theta):
# 计算散射光子能量和波长
E_1 = E_0 / (1 + E_0 / (m_e * c ** 2) * (1 - np.cos(theta)))
lambda_1 = h * c / E_1
# 计算散射角度
phi = np.random.uniform(0, 2 * np.pi)
theta_1 = np.arccos((E_0 + m_e * c ** 2) / (E_1 + m_e * c ** 2) * (1 - np.cos(theta))) + phi
return lambda_1
# 模拟散射过程
n = 100000
theta = np.random.uniform(0, np.pi, n)
lambda_1 = np.array([compton_scatter(t) for t in theta])
# 绘制结果
plt.hist(lambda_1 * 1e10, bins=100)
plt.xlabel('Wavelength (Angstrom)')
plt.ylabel('Counts')
plt.title('Compton Scattering Simulation')
plt.show()
```
该程序首先定义了一些常量,包括电子质量、光速、普朗克常数、入射光波长和入射光子能量。然后定义了一个函数`compton_scatter`,用于计算散射光子的能量和波长。最后,程序模拟了100000次散射过程,并绘制了散射光子波长的直方图。
相关推荐
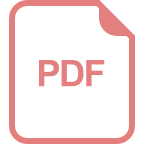
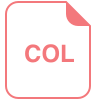













