完整的java代码演示
时间: 2024-12-18 12:13:46 浏览: 6
在Java中,完整的代码演示通常需要根据特定的需求来编写,这里我将给出一个简单的例子,展示如何使用Netty构建一个基础的TCP客户端,它具备自动重连功能:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class NettyAutoReconnectClient {
private static final int PORT = 8080;
private static final int MAX_RETRIES = 5;
private static final long RECONNECT_INTERVAL = 5000; // 每次重连间隔5秒
public static void main(String[] args) throws Exception {
EventLoopGroup group = new NioEventLoopGroup(); // 创建事件循环组
try {
Bootstrap bootstrap = new Bootstrap(); // 创建启动器
bootstrap.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() { // 初始化管道
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ReconnectingHandler());
}
});
for (int i = 0; i < MAX_RETRIES; i++) {
System.out.println("Connecting attempt " + (i + 1));
ChannelFuture future = bootstrap.connect("localhost", PORT); // 连接服务器
future.channel().eventLoop().schedule(() -> {
future.channel().close().sync(); // 如果连接失败,主动关闭并重试
if (future.isSuccess()) {
break; // 如果成功,退出循环
}
scheduleReconnect();
}, RECONNECT_INTERVAL, TimeUnit.MILLISECONDS);
}
future.channel().closeFuture().sync(); // 等待所有操作完成
} finally {
group.shutdownGracefully(); // 关闭事件循环组
}
}
private static void scheduleReconnect() {
new Thread(() -> {
try {
Thread.sleep(RECONNECT_INTERVAL);
} catch (InterruptedException e) {
e.printStackTrace();
}
main(null);
}).start();
}
}
class ReconnectingHandler extends ChannelInboundHandlerAdapter {
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close(); // 发生错误,关闭连接
}
}
```
这个示例中,`ReconnectingHandler`负责处理异常,当发生异常时关闭当前通道。`main`方法里,我们创建了一个Bootstrap实例,设置连接参数,并在一个循环中尝试连接,如果连接失败,会延迟一定时间再尝试,直到达到最大重试次数。
阅读全文
相关推荐




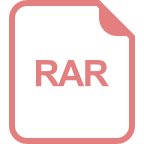
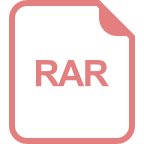




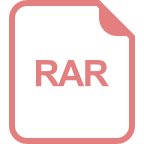
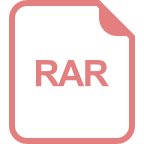


