python GPU计时
时间: 2023-09-27 17:03:58 浏览: 165
如果想在Python中计时GPU运算的时间,可以使用如下代码:
```python
import time
import tensorflow as tf
# 创建一个用于测试的随机数组
x = tf.random.normal((1000, 1000))
# 创建一个GPU设备
device_name = tf.test.gpu_device_name()
if device_name != '/device:GPU:0':
raise SystemError('GPU device not found')
print('Found GPU at: {}'.format(device_name))
# 开始计时
start_time = time.time()
# 进行GPU运算
with tf.device('/device:GPU:0'):
for i in range(100):
x = tf.matmul(x, x)
# 计算时间
end_time = time.time()
print('Time taken: {} seconds'.format(end_time - start_time))
```
这段代码首先创建了一个用于测试的随机数组 `x`,然后创建了一个GPU设备。接着,使用 `with tf.device('/device:GPU:0')` 来指定GPU设备进行运算。最后,使用 `time.time()` 函数计算运算时间。运行代码后,会输出GPU设备信息和运算时间。
相关问题
pythonGPU双精度算力测试代码
在Python中,如果你想要测试GPU的双精度浮点数(通常指单精度为float64)计算能力,你可以利用深度学习库如PyTorch或TensorFlow提供的功能。下面是一个简单的例子,使用PyTorch:
```python
import torch
# 检查可用的GPU设备及其CUDA版本
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Running on {device}: CUDA version {torch.version.cuda}")
# 创建一个大的张量并将其移动到GPU上
large_tensor = torch.randn(1024, 1024).double().to(device)
start_time = torch.cuda.Event(enable_timing=True)
end_time = torch.cuda.Event(enable_timing=True)
# 执行一次双精度浮点运算,例如矩阵乘法
with torch.no_grad():
start_time.record()
result = torch.matmul(large_tensor, large_tensor.t())
end_time.record()
# 等待所有事件完成计时
torch.cuda.synchronize()
# 计算时间
time_taken = start_time.elapsed_time(end_time) / 1e3
print(f"Time taken for a double precision matrix multiplication: {time_taken:.6f} ms")
python代码测试GPU单精度算力
在Python中,你可以使用第三方库如`tensorflow`、`pytorch`或者`cupy`来测试GPU的单精度浮点运算性能。这里以`tensorflow`为例:
首先,确保已经安装了`tensorflow`,如果没有,可以使用pip安装:
```bash
pip install tensorflow
```
然后,你可以编写一段简单的代码来测量GPU上单精度(float32)运算的性能。例如,计算矩阵乘法就是一个常见的测试操作:
```python
import tensorflow as tf
# 创建两个随机生成的大张量
x = tf.random.normal(shape=(1000, 1000), dtype=tf.float32)
y = tf.random.normal(shape=(1000, 1000), dtype=tf.float32)
# 定义GPU加速的矩阵乘法函数
@tf.function(device='GPU')
def matmul_gpu(x, y):
return tf.matmul(x, y)
# 开始计时
start_time = time.time()
result_gpu = matmul_gpu(x, y)
end_time = time.time()
# 计算并打印单精度浮点运算时间
gpu_time = end_time - start_time
print(f"GPU单精度矩阵乘法的时间: {gpu_time}秒")
阅读全文
相关推荐
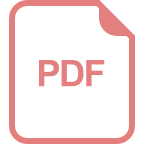
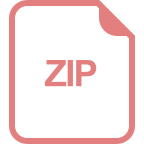
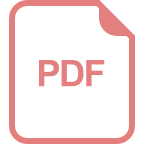
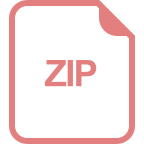
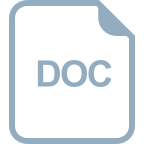
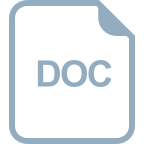
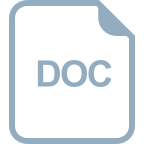
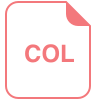
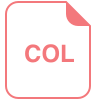
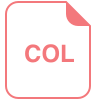
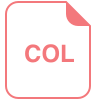
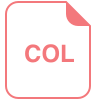
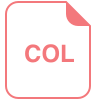
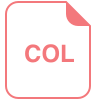
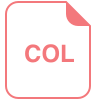