如何进行用户的身份验证
时间: 2024-04-01 18:37:10 浏览: 16
在 .NET Core 中,可以使用身份验证来对用户进行身份验证。具体实现步骤如下:
1. 在 Startup.cs 文件中,添加身份验证服务。
```csharp
public void ConfigureServices(IServiceCollection services)
{
// 添加身份验证服务
services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme)
.AddCookie(options =>
{
options.LoginPath = "/Account/Login";
});
// 添加其他服务
services.AddMvc();
}
```
以上代码中,我们使用 AddAuthentication 方法添加身份验证服务,并指定了使用 CookieAuthenticationDefaults.AuthenticationScheme 方案。另外,我们还使用 AddCookie 方法添加了 Cookie 认证方案,并指定了登录路径为 /Account/Login。
2. 在 AccountController.cs 文件中,添加登录和注销方法。
```csharp
public class AccountController : Controller
{
[HttpGet]
public IActionResult Login()
{
return View();
}
[HttpPost]
public async Task<IActionResult> Login(LoginViewModel model)
{
if (ModelState.IsValid)
{
// 进行身份验证
var identity = new ClaimsIdentity(CookieAuthenticationDefaults.AuthenticationScheme);
identity.AddClaim(new Claim(ClaimTypes.Name, model.UserName));
identity.AddClaim(new Claim(ClaimTypes.Role, "User"));
var principal = new ClaimsPrincipal(identity);
await HttpContext.SignInAsync(CookieAuthenticationDefaults.AuthenticationScheme, principal);
// 跳转到首页
return RedirectToAction("Index", "Home");
}
return View(model);
}
[HttpGet]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync(CookieAuthenticationDefaults.AuthenticationScheme);
return RedirectToAction("Index", "Home");
}
}
```
以上代码中,我们定义了 Login 和 Logout 方法。在 Login 方法中,我们使用 ClaimsIdentity 和 ClaimsPrincipal 类来创建用户身份信息,并使用 HttpContext.SignInAsync 方法将用户身份信息保存到 Cookie 中。在 Logout 方法中,我们使用 HttpContext.SignOutAsync 方法来清除用户登录信息。
3. 在需要进行身份验证的控制器或方法中,添加 [Authorize] 标记。
```csharp
[Authorize(Roles = "User")]
public class UserController : Controller
{
// ...
}
```
以上代码中,我们使用 [Authorize(Roles = "User")] 标记来表示该控制器或方法需要对用户进行身份验证,并且用户必须属于 "User" 角色。
这样就可以通过身份验证来对用户进行身份验证了。在需要进行身份验证的控制器或方法中,如果用户没有通过身份验证,则会被自动重定向到登录页面。
相关推荐
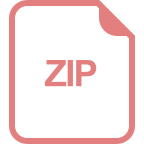














