1,用C语言的库函数实现cat命令 2,库函数实现一个文件中每个字符的个数,在Ubuntu上运行
时间: 2024-12-12 18:22:52 浏览: 18
1. 使用C语言的库函数实现cat命令类似地,可以利用标准输入输出流(stdio.h库)和文件操作功能来模拟这个功能。下面是一个简单的示例,它打开指定的文件并逐行读取内容到标准输出:
```c
#include <stdio.h>
#include <stdlib.h>
void cat_file(const char* filename) {
FILE* file = fopen(filename, "r"); // 打开文件
if (file == NULL) {
perror("Error opening file");
exit(EXIT_FAILURE);
}
int c;
while ((c = fgetc(file)) != EOF) { // 遍历文件直到末尾
printf("%c", c); // 输出每个字符
}
fclose(file); // 关闭文件
}
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <filename>\n", argv[0]);
return EXIT_FAILURE;
}
cat_file(argv[1]); // 调用函数处理参数
return 0;
}
```
要在Ubuntu上运行这个程序,你需要先创建一个`.c`源文件(如`catimpl.c`),然后编译并运行:
```sh
gcc -o catimpl catimpl.c
./catimpl /path/to/your/file.txt
```
2. 实现统计文件中每个字符的个数,可以稍微修改上面的cat_file函数,增加一个计数器来记录字符数:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的函数
int count_chars(const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
int total_chars = 0;
int c;
while ((c = fgetc(file)) != EOF) {
total_chars += isprint(c); // 计算可打印字符(包括空格、字母等)
}
fclose(file);
return total_chars;
}
int main() {
if (argc != 2) {
// ...
}
int chars_count = count_chars(argv[1]);
printf("Total characters in the file: %d\n", chars_count);
return 0;
}
```
运行时同样需要提供文件路径作为命令行参数。
阅读全文
相关推荐



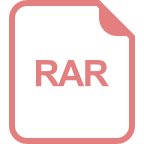
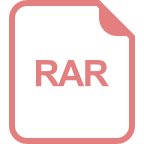
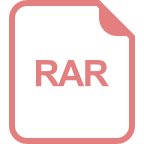
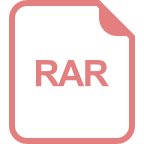

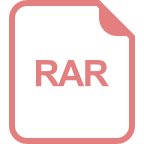
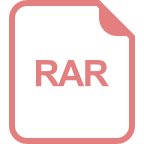
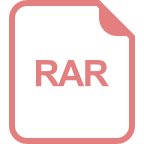


