labwindows cvi 中如何实现线程锁
时间: 2023-09-18 12:14:37 浏览: 72
在 LabWindows/CVI 中实现线程锁通常需要使用互斥量(Mutex)来控制共享资源的访问。具体步骤如下:
1. 创建一个互斥量对象,使用 Mutex New() 函数进行创建。
2. 在需要访问共享资源的线程中,使用 Mutex Lock() 函数对互斥量进行加锁,以确保只有一个线程能够访问共享资源。
3. 当线程访问完共享资源后,使用 Mutex Unlock() 函数将互斥量解锁,以允许其他线程访问共享资源。
需要注意的是,在使用互斥量的过程中,需要确保所有访问共享资源的线程都使用同一个互斥量对象,否则可能会引起死锁等问题。同时,互斥量并不能保证多线程程序的正确性,还需要考虑其他因素,如竞态条件等。
示例代码如下:
```
#include <cvirte.h>
#include <userint.h>
#include <ansi_c.h>
#include <pthread.h>
static pthread_mutex_t mutex;
int CVICALLBACK ThreadFunction(void *functionData)
{
while(1)
{
// 加锁
pthread_mutex_lock(&mutex);
// 访问共享资源
printf("Thread 1 is accessing shared resource.\n");
// 解锁
pthread_mutex_unlock(&mutex);
}
}
int main(int argc, char *argv[])
{
// 创建互斥量对象
pthread_mutex_init(&mutex, NULL);
// 创建线程
pthread_t thread;
pthread_create(&thread, NULL, ThreadFunction, NULL);
while(1)
{
// 加锁
pthread_mutex_lock(&mutex);
// 访问共享资源
printf("Main thread is accessing shared resource.\n");
// 解锁
pthread_mutex_unlock(&mutex);
}
// 销毁互斥量对象
pthread_mutex_destroy(&mutex);
return 0;
}
```
相关推荐
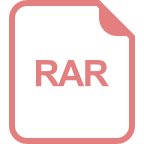














