python读取config.properties文件的值
时间: 2024-02-03 08:15:05 浏览: 134
假设你的`config.properties`文件如下:
```
db.host=localhost
db.port=3306
db.username=root
db.password=123456
```
你可以使用Python的ConfigParser模块来读取这个文件中的值。下面是一个示例代码:
```python
import configparser
config = configparser.ConfigParser()
config.read('config.properties')
db_host = config.get('db', 'host')
db_port = config.getint('db', 'port')
db_username = config.get('db', 'username')
db_password = config.get('db', 'password')
print(db_host, db_port, db_username, db_password)
```
输出结果为:
```
localhost 3306 root 123456
```
这里的`get`方法用于获取字符串类型的值,`getint`方法用于获取整数类型的值。`'db'`表示读取配置文件中`[db]`段中的值。`'host'`、`'port'`、`'username'`、`'password'`是这个段中的键。
相关问题
python如何读取config.properties里面的配置项
可以使用Python的ConfigParser模块来读取config.properties文件中的配置项。以下是一个示例代码:
```python
import configparser
config = configparser.ConfigParser()
config.read('config.properties')
# 读取单个配置项
value = config.get('section_name', 'property_name')
# 读取整个section的配置项
section = config['section_name']
```
需要注意的是,ConfigParser模块只能读取以“键-值”形式存储的配置文件(如config.properties),如果配置文件中有其他格式,需要使用其他模块进行解析。
python2,已知配置文件路径/opt/hikvision/web/opsMgrCenter/conf和名称config.properties,如何读取文件中包含machine.ip的这一行数据
对于Python 2,你可以使用以下代码来读取/opt/hikvision/web/opsMgrCenter/conf/config.properties文件中包含'machine.ip'字符串的行:
```python
with open('/opt/hikvision/web/opsMgrCenter/conf/config.properties', 'r') as f:
for line in f:
if 'machine.ip' in line:
print(line)
```
这段代码与Python 3中的代码基本相同,只是在打开文件时需要使用完整的文件路径,并且字符串的拼接方式略有不同。在Python 2中,你可以使用字符串连接符号 + 来拼接路径和文件名,如下所示:
```python
with open('/opt/hikvision/web/opsMgrCenter/conf/' + 'config.properties', 'r') as f:
for line in f:
if 'machine.ip' in line:
print(line)
```
请注意,上述代码假定/opt/hikvision/web/opsMgrCenter/conf目录和config.properties文件均存在,并且文件中确实包含'machine.ip'字符串。如果文件不存在或不包含该字符串,代码可能会出现错误。
阅读全文
相关推荐










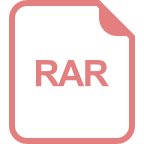



