uniapp开发的APP页面如何设置deeplink URL代码实现
时间: 2023-05-31 18:03:57 浏览: 669
要设置deeplink URL,可以按照以下步骤进行操作:
1. 在manifest.json文件中,添加"app-plus"键,值为一个对象,其中包含"launch_path"键,值为你想要设置的deeplink URL路径。例如:
```
"app-plus": {
"launch_path": "/myapp/deeplink"
}
```
2. 在uni-app中,可以使用uni.navigateTo和uni.redirectTo方法来跳转到deeplink URL。例如:
```
uni.navigateTo({
url: '/myapp/deeplink'
});
```
3. 在webview中,可以通过location.href属性来跳转到deeplink URL。例如:
```
location.href = 'myapp://deeplink';
```
需要注意的是,deeplink URL需要和APP中的路由设置保持一致,否则跳转可能会失败。另外,在使用deeplink URL时,需要保证APP已经被安装并处于运行状态。
相关问题
APP页面如何设置deeplink URL代码实现
在APP页面中设置deeplink URL需要在AndroidManifest.xml文件中添加以下代码:
```xml
<activity android:name=".YourActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data
android:host="yourapp.com"
android:pathPrefix="/yourpath"
android:scheme="yourapp" />
</intent-filter>
</activity>
```
其中,android:host表示你的应用程序的主机名,android:pathPrefix表示你的URI路径的前缀,android:scheme表示你的URI方案。
在iOS中,需要在Info.plist文件中添加以下代码:
```xml
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLSchemes</key>
<array>
<string>yourapp</string>
</array>
<key>CFBundleURLName</key>
<string>yourapp</string>
</dict>
</array>
```
其中,CFBundleURLSchemes表示你的URI方案,CFBundleURLName表示你的应用程序的名称。
通过以上设置,你的应用程序就可以响应deeplink URL了。例如,你可以在浏览器中输入“yourapp://yourpath”来打开你的应用程序并跳转到指定页面。
uniapp开发app一键登录流程,代码如何实现
实现uniapp开发app的一键登录流程,需要涉及到前端和后端两部分代码实现。
前端代码实现:
1. 在uniapp的登录页面中,添加一键登录按钮。
2. 点击一键登录按钮时,调用uniapp提供的API,获取用户的手机号码,并将手机号码发送给后端服务器。
```
<template>
<view>
<button @click="oneClickLogin">一键登录</button>
</view>
</template>
<script>
export default {
methods: {
oneClickLogin() {
uni.login({
provider: 'univerify',
success: res => {
uni.request({
url: 'https://your-backend-server-url.com/oneClickLogin',
method: 'POST',
data: {
phone: res.authResult.phoneNumber
},
success: res => {
// 登录成功,保存token
uni.setStorageSync('token', res.data.token)
// 跳转到主页
uni.switchTab({
url: '/pages/home/home'
})
},
fail: err => {
console.log(err)
}
})
},
fail: err => {
console.log(err)
}
})
}
}
}
</script>
```
后端代码实现:
1. 接收前端发送的手机号码数据。
2. 向运营商发送请求,获取用户的手机号码和验证码。
3. 验证用户的验证码,如果正确则生成一个token并返回给前端。
```
app.post('/oneClickLogin', (req, res) => {
const { phone } = req.body
// 向运营商发送请求,获取用户的手机号码和验证码
// ...
// 验证用户的验证码是否正确
if (verifyCode === '123456') {
// 生成token
const token = jwt.sign({ phone }, 'secret', { expiresIn: '1h' })
// 返回token
res.json({
code: 200,
message: '登录成功',
token
})
} else {
res.json({
code: 401,
message: '验证码错误'
})
}
})
```
需要注意的是,以上代码仅供参考,实际开发中还需要进行详细的测试和调试,以保证应用的正常运行。同时,还需要考虑到用户的手机号码的准确性和安全性,以及数据加密和token安全等问题,以保证应用的安全性。
阅读全文
相关推荐
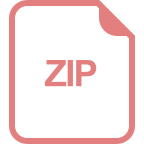
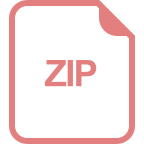
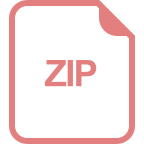













